Ignite UI API Reference
ig.KnockoutDataSource
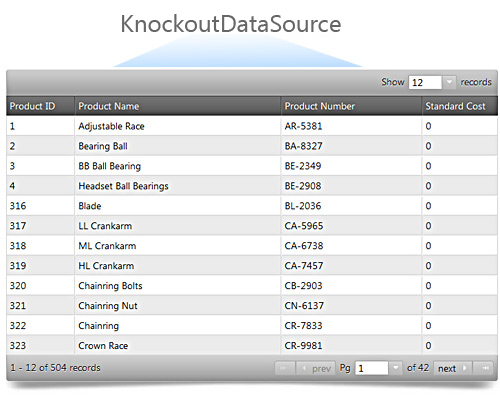
The KnockoutDataSource extends the DataSource class and is a client-side component which adds Knockout.js support for both Ignite UI controls as well as plain DOM elements. The KnockoutDataSource allows you to synchronize your page’s model and views by creating bindings for adding, updating and deleting rows. Also data binding (reading data) is completely transparent, meaning you can use an observable array as a data store and it works seamlessly with knockout.js requiring no extra code. Both arrays of rows, as well as individual cells can set up as observable objects. The data source features support for filtering, paging, sorting, column summaries and updating data. Further information regarding the classes, options, events, methods and themes of this API are available under the associated tabs above.
Code Sample
<!doctype html> <html> <head> <!-- jQuery Core --> <script src="js/jquery.js" type="text/javascript"></script> <!-- jQuery UI --> <script src="js/jquery-ui.js" type="text/javascript"></script> <!-- Infragistics Loader Script --> <script src="js/infragistics.loader.js" type="text/javascript"></script> <script src="js/adventureWorks.min.js" type="text/javascript"></script> <!-- Infragistics Loader Initialization --> <script type="text/javascript"> var itemsModel, db = [{"Name": "Bread","Rating": 4,"Price": "2.5"}, {"Name": "Milk", "Rating": 3, "Price": "3.5"}, {"Name": "Vint soda", "Rating": 3, "Price": "20.9"}]; function Item(name, price, rating) { return { name: ko.observable(name), price: ko.observable(price), rating: ko.observable(rating) }; }; function ItemsViewModel() { var self = this; self.data = ko.observableArray([ ]); for (var i = 0; i < 3; i++) { self.data.push(new Item(db[i].Name, db[i].Price, db[i].Rating)); } } var ds, render = function (success, error) { if (success) { ds.addRow(4, {name : "CD Player", price : "40", rating : 4}, true); $($.ig.tmpl("<tr><td>Name: ${name}</td><td>Price: ${price}</td><td>Rating: ${rating}</td></tr>", ds.dataView())).appendTo("#table1"); } else { alert(error); } } $.ig.loader(function () { var model = new ItemsViewModel(); ko.applyBindings(model); ds = new $.ig.KnockoutDataSource({ callback: render, dataSource: model, type: 'json', responseDataKey: 'data' }); ds.dataBind(); }); </script> </head> <body> <table id="table1"></table> </body> </html>
Dependencies
jquery-1.7.1.js
ig.util.js
ig.datasource.js
The current widget has no options.
The current widget has no events.
-
- new $.ig.KnockoutDataSource( options:object );
- options
- Type:object
Code Sample
var itemsModel, db = [{"Name": "Bread","Rating": 4,"Price": "2.5"}, {"Name": "Milk", "Rating": 3, "Price": "3.5"}, {"Name": "Vint soda", "Rating": 3, "Price": "20.9"}]; function Item(name, price, rating) { return { name: ko.observable(name), price: ko.observable(price), rating: ko.observable(rating) }; }; function ItemsViewModel() { var self = this; self.data = ko.observableArray([ ]); for (var i = 0; i < 3; i++) { self.data.push(new Item(db[i].Name, db[i].Price, db[i].Rating)); } } var ds, render = function (success, error) { if (success) { $($.ig.tmpl("<tr><td>Name: ${name}</td><td>Price: ${Price}</td><td>Rating: ${Rating}</td></tr>", ds.dataView())).appendTo("#table1"); } else { alert(error); } } $.ig.loader(function () { var model = new ItemsViewModel(); ko.applyBindings(model); ds = new $.ig.KnockoutDataSource({ callback: render, dataSource: model, type: 'json', responseDataKey: 'data' }); ds.dataBind(); });
-
addRow
- .addRow( rowId:object, rowObject:object, autoCommit:object );
- rowId
- Type:object
- rowObject
- Type:object
- autoCommit
- Type:object
Code Sample
var itemsModel, db = [{"Name": "Bread","Rating": 4,"Price": "2.5"}, {"Name": "Milk", "Rating": 3, "Price": "3.5"}, {"Name": "Vint soda", "Rating": 3, "Price": "20.9"}]; function Item(name, price, rating) { return { name: ko.observable(name), price: ko.observable(price), rating: ko.observable(rating) }; }; function ItemsViewModel() { var self = this; self.data = ko.observableArray([ ]); for (var i = 0; i < 3; i++) { self.data.push(new Item(db[i].Name, db[i].Price, db[i].Rating)); } } var ds, render = function (success, error) { if (success) { //$("#table1 tbody").empty(); ds.addRow(4, {name : "CD Player", price : "40", rating : 4}, true); $($.ig.tmpl("<tr><td>Name: ${name}</td><td>Price: ${Price}</td><td>Rating: ${Rating}</td></tr>", ds.dataView())).appendTo("#table1"); } else { alert(error); } } $.ig.loader(function () { var model = new ItemsViewModel(); ko.applyBindings(model); ds = new $.ig.KnockoutDataSource({ callback: render, dataSource: model, type: 'json', responseDataKey: 'data' }); ds.dataBind(); });
-
dataAt
- .dataAt( path:object, keyspath:object );
- path
- Type:object
- keyspath
- Type:object
-
deleteRow
- .deleteRow( rowId:object, autoCommit:object );
- rowId
- Type:object
- autoCommit
- Type:object
Code Sample
var itemsModel, db = [{"Name": "Bread","Rating": 4,"Price": "2.5"}, {"Name": "Milk", "Rating": 3, "Price": "3.5"}, {"Name": "Vint soda", "Rating": 3, "Price": "20.9"}]; function Item(name, price, rating) { return { name: ko.observable(name), price: ko.observable(price), rating: ko.observable(rating) }; }; function ItemsViewModel() { var self = this; self.data = ko.observableArray([ ]); for (var i = 0; i < 3; i++) { self.data.push(new Item(db[i].Name, db[i].Price, db[i].Rating)); } } var ds, render = function (success, error) { if (success) { ds.deleteRow(0, true); $($.ig.tmpl("<tr><td>Name: ${name}</td><td>Price: ${Price}</td><td>Rating: ${Rating}</td></tr>", ds.dataView())).appendTo("#table1"); } else { alert(error); } } $.ig.loader(function () { var model = new ItemsViewModel(); ko.applyBindings(model); ds = new $.ig.KnockoutDataSource({ callback: render, dataSource: model, type: 'json', responseDataKey: 'data' }); ds.dataBind(); });
-
setCellValue
- .setCellValue( rowId:object, colId:object, val:object, autoCommit:object );
- rowId
- Type:object
- colId
- Type:object
- val
- Type:object
- autoCommit
- Type:object
Code Sample
var itemsModel, db = [{"Name": "Bread","Rating": 4,"Price": "2.5"}, {"Name": "Milk", "Rating": 3, "Price": "3.5"}, {"Name": "Vint soda", "Rating": 3, "Price": "20.9"}]; function Item(name, price, rating) { return { name: ko.observable(name), price: ko.observable(price), rating: ko.observable(rating) }; }; function ItemsViewModel() { var self = this; self.data = ko.observableArray([ ]); for (var i = 0; i < 3; i++) { self.data.push(new Item(db[i].Name, db[i].Price, db[i].Rating)); } } var ds, render = function (success, error) { if (success) { ds.setCellValue(1, "Name", "DVD Player", true); $($.ig.tmpl("<tr><td>Name: ${name}</td><td>Price: ${Price}</td><td>Rating: ${Rating}</td></tr>", ds.dataView())).appendTo("#table1"); } else { alert(error); } } $.ig.loader(function () { var model = new ItemsViewModel(); ko.applyBindings(model); ds = new $.ig.KnockoutDataSource({ callback: render, dataSource: model, type: 'json', responseDataKey: 'data' }); ds.dataBind(); });
-
updateRow
- .updateRow( rowId:object, rowObject:object, autoCommit:object );
- rowId
- Type:object
- rowObject
- Type:object
- autoCommit
- Type:object
Code Sample
var itemsModel, db = [{"Name": "Bread","Rating": 4,"Price": "2.5"}, {"Name": "Milk", "Rating": 3, "Price": "3.5"}, {"Name": "Vint soda", "Rating": 3, "Price": "20.9"}]; function Item(name, price, rating) { return { name: ko.observable(name), price: ko.observable(price), rating: ko.observable(rating) }; }; function ItemsViewModel() { var self = this; self.data = ko.observableArray([ ]); for (var i = 0; i < 3; i++) { self.data.push(new Item(db[i].Name, db[i].Price, db[i].Rating)); } } var ds, render = function (success, error) { if (success) { ds.updateRow(1, { Name: "DVD Player1", Price: "10", Rating: "5" }, true); $($.ig.tmpl("<tr><td>Name: ${name}</td><td>Price: ${Price}</td><td>Rating: ${Rating}</td></tr>", ds.dataView())).appendTo("#table1"); } else { alert(error); } } $.ig.loader(function () { var model = new ItemsViewModel(); ko.applyBindings(model); ds = new $.ig.KnockoutDataSource({ callback: render, dataSource: model, type: 'json', responseDataKey: 'data' }); ds.dataBind(); });