Ignite UI API Reference
ui.igMap
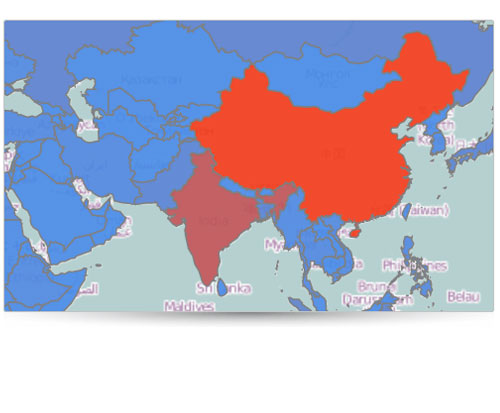
The igMap control is a HTML5 jQuery map control based on the Infragistics Silverlight xamMap™ control. The control plots generic map data from map providers (e.g. OpenStreetMap®, Bing® Maps, and CloudMade® Maps) and exposes the ability to render additional map layers. For example, you can mark specific map locations and display information on the map point, you can use shape files to draw shapes around geographical regions and use differing colors depending on data parameters attached to map regions. Further information regarding the classes, options, events, methods and themes of this API are available under the associated tabs above.
The following code snippet demonstrates how to initialize the igMap control.
For details on how to reference the required scripts and themes for the igMap control read, Using JavaScript Resources in Ignite UI and Styling and Theming Ignite UI.
Code Sample
<!doctype html> <html> <head> <title>Ignite UI igMap</title> <!-- jQuery Core --> <script src="js/jquery.js" type="text/javascript"></script> <!-- jQuery UI --> <script src="js/jquery-ui.js" type="text/javascript"></script> <!-- Infragistics Loader --> <script type="text/javascript" src="http://localhost/ig_ui/js/infragistics.loader.js"></script> <script type="text/javascript"> var data = [ { Name: "London", Country: "England", Latitude: 51.50, Longitude: 0.12 }, { Name: "Berlin", Country: "Germany", Latitude: 52.50, Longitude: 13.33 }, { Name: "Moscow", Country: "Russia", Latitude: 55.75, Longitude: 37.51 }, { Name: "Sydney", Country: "Australia", Latitude: -33.83, Longitude: 151.2 }, { Name: "Tokyo", Country: "Japan", Latitude: 35.6895, Longitude: 139.6917 }, { Name: "Shanghai", Country: "China", Latitude: 31.2244, Longitude: 121.4759 }, { Name: "New York", Country: "United States", Latitude: 40.7561, Longitude: -73.9870 }, { Name: "Sao Paulo", Country: "Brasil", Latitude: -23.5489, Longitude: -46.6388 }, { Name: "Los Angeles", Country: "United States", Latitude: 34.0522, Longitude: -118.2434 } ]; </script> <script id="cityTemplate" type="text/x-jquery-tmpl"> <table> <tr><td>${item.Name}, ${item.Country}</td></tr> <tr><td>Latitude: ${item.Latitude}</td></tr> <tr><td>Longitude: ${item.Longitude}</td></tr> </table> </script> <script type="text/javascript"> $.ig.loader({ scriptPath: "http://localhost/ig_ui/js/", cssPath: "http://localhost/ig_ui/css/", resources: "igMap" }); $.ig.loader(function () { $("#map").igMap({ width: "100%", verticalZoomable: true, horizontalZoomable: true, overviewPlusDetailPaneVisibility: "visible", backgroundContent: { type: "openStreet" }, series: [{ type: "geographicSymbol", name: "worldCities", dataSource: data, latitudeMemberPath: "Latitude", longitudeMemberPath: "Longitude", markerType: "automatic", markerBrush: "olive", showTooltip: true, tooltipTemplate: "cityTemplate" }], windowResponse: "immediate", windowRect: { left: 0.20, top: 0.20, height: 0.45, width: 0.45 } }); }); </script> </head> <body> <div id="map"></div> </body> </html>
Related Samples
Dependencies
Inherits
-
autoMarginHeight
- Type:
- number
- Default:
- 0
Sets or gets the automatic height to add when automatically adding margins to the map.
Code Sample
//Initialize $(".selector").igMap({ autoMarginHeight : 100 }); //Get var height = $(".selector").igMap("option", "autoMarginHeight"); //Set $(".selector").igMap("option", "autoMarginHeight", 100);
-
autoMarginWidth
- Type:
- number
- Default:
- 20
Sets or gets the automatic width to add when automatically adding margins to the map.
Code Sample
//Initialize $(".selector").igMap({ autoMarginWidth : 200 }); //Get var width = $(".selector").igMap("option", "autoMarginWidth"); //Set $(".selector").igMap("option", "autoMarginWidth", 200);
-
backgroundContent
- Type:
- object
- Default:
- {}
Background content object.
Code Sample
//Initialization $("#map").igMap({ backgroundContent: { type: "bing", key: "abcdefghijk123456" } }); //Get var backgroundContent = $(".selector").igMap("option", "backgroundContent"); //Set $(".selector").igMap("option", "backgroundContent", { type: "bing", key: "abcdefghijk123456" });
-
bingUrl
- Type:
- string
- Default:
- http://dev.virtualearth.net/rest/v1/imagery/metadata/
Gets or sets the bing maps url.
Code Sample
//Initialization $("#map").igMap({ backgroundContent: { type: "bing", key: "abcdefghijk123456", bingUrl: "http://dev.virtualearth.net/rest/v1/imagery/metadata/" } }); //Get var backgroundContent = $(".selector").igMap("option", "backgroundContent"); backgroundContent.bingUrl; //Set $(".selector").igMap("option", "backgroundContent", { type: "bing", key: "abcdefghijk123456", bingUrl: "http://dev.virtualearth.net/rest/v1/imagery/metadata/" });
-
imagerySet
- Type:
- string
- Default:
- aerialwithlabels
Gets or sets the type of the imagery.
Code Sample
//Initialization $("#map").igMap({ backgroundContent: { type: "bing", key: "abcdefghijk123456", imagerySet: "aerial" } }); //Get var backgroundContent = $(".selector").igMap("option", "backgroundContent"); backgroundContent.imagerySet; //Set $(".selector").igMap("option", "backgroundContent", { type: "bing", key: "abcdefghijk123456", imagerySet: "aerial" });
-
key
- Type:
- string
- Default:
- null
Gets or sets the key.
Code Sample
//Initialization $("#map").igMap({ backgroundContent: { type: "bing", key: "abcdefghijk123456" } }); //Get var backgroundContent = $(".selector").igMap("option", "backgroundContent"); backgroundContent.key; //Set $(".selector").igMap("option", "backgroundContent", { type: "bing", key: "abcdefghijk123456" });
-
parameter
- Type:
- string
- Default:
- null
Gets or sets the parameter.
Code Sample
//Initialization $("#map").igMap({ backgroundContent: { type: "bing", key: "abcdefghijk123456", parameter: 2 } }); //Get var backgroundContent = $(".selector").igMap("option", "backgroundContent"); backgroundContent.parameter; //Set $(".selector").igMap("option", "backgroundContent", { type: "bing", key: "abcdefghijk123456", parameter: 2 });
-
tilePath
- Type:
- string
- Default:
- null
Gets or sets the map tile image uri.
Code Sample
//Initialization $("#map").igMap({ backgroundContent: { type: "bing", key: "abcdefghijk123456", tilePath: "http://www.example.com/tiles/" } }); //Get var backgroundContent = $(".selector").igMap("option", "backgroundContent"); backgroundContent.tilePath; //Set $(".selector").igMap("option", "backgroundContent", { type: "bing", key: "abcdefghijk123456", tilePath: "http://www.example.com/tiles/" });
-
type
- Type:
- enumeration
- Default:
- openstreet
Type of the background content for the map.
Members
- openStreet
- Type:string
- Specify the background content to display OpenStreetMap geographic data. Set as default.
- cloudMade
- Type:string
- Specify the background content to display CloudMade geographic data.
- bing
- Type:string
- Specify the background content to BingMaps geographic data.
Code Sample
//Initialization $("#map").igMap({ backgroundContent: { type: "bing", key: "abcdefghijk123456" } }); //Get var backgroundContent = $(".selector").igMap("option", "backgroundContent"); backgroundContent.type; //Set $(".selector").igMap("option", "backgroundContent", { type: "bing", key: "abcdefghijk123456" });
-
circleMarkerTemplate
- Type:
- object
- Default:
- null
Gets or sets the template to use for circle markers on the map.
Defines the marker template used for
series with a marker type of circle.
The provided object should have properties called render and optionally measure. See definition for option: legendItemBadgeTemplate.Code Sample
//Initialize $(".selector").igMap({ circleMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
crosshairPoint
- Type:
- object
- Default:
- {}
Gets or sets the cross hair point (in world coordinates)
Either or both of the crosshair point's X and Y may be set to double.NaN, in which
case the relevant crosshair line is hidden.Code Sample
// Initialization $(".selector").igMap({ crosshairPoint: { x: 0.5, y: 0.5 } }); // Get var crosshairPoint = $(".selector").igMap("option", "crosshairPoint"); // Set $(".selector").igMap("option", "crosshairPoint", { x: 0.5, y: 0.5 });
-
x
- Type:
- number
- Default:
- ""
The x coordinate.
Code Sample
// Initialization $(".selector").igMap({ crosshairPoint: { x: 0.5, y: 0.5 } }); // Get var crosshairPoint = $(".selector").igMap("option", "crosshairPoint"); var x = crosshairPoint.x; // Set $(".selector").igMap("option", "crosshairPoint", { x: 0.5, y: 0.5 });
-
y
- Type:
- number
- Default:
- ""
The y coordinate.
Code Sample
// Initialization $(".selector").igMap({ crosshairPoint: { x: 0.5, y: 0.5 } }); // Get var crosshairPoint = $(".selector").igMap("option", "crosshairPoint"); var y = crosshairPoint.y; // Set $(".selector").igMap("option", "crosshairPoint", { x: 0.5, y: 0.5 });
-
crosshairVisibility
- Type:
- enumeration
- Default:
- collapsed
Gets or sets the current Map's crosshair visibility override.
Members
- visible
- Type:string
- Crosshair should be visible.
- collapsed
- Type:string
- Crosshair should not be visible.
Code Sample
// Initialization $(".selector").igMap({ crosshairVisibility: "collapsed" }); // Get var crosshairVisibility = $(".selector").igMap("option", "crosshairVisibility"); // Set $(".selector").igMap("option", "crosshairVisibility", "collapsed");
-
dataSource
- Type:
- object
- Default:
- null
Can be any valid data source accepted by $.ig.DataSource, or an instance of an $.ig.DataSource itself.
Code Sample
// Initialization var data = [ { Name: "London", Country: "England", Latitude: 51.50, Longitude: 0.12 }, { Name: "Berlin", Country: "Germany", Latitude: 52.50, Longitude: 13.33 }, { Name: "Moscow", Country: "Russia", Latitude: 55.75, Longitude: 37.51 }, { Name: "Sydney", Country: "Australia", Latitude: -33.83, Longitude: 151.2 } ]; $(".selector").igMap({ dataSource: data }); // Get var dataSource = $(".selector").igMap("option", "dataSource"); // Set $(".selector").igMap("option", "dataSource", data);
-
dataSourceType
- Type:
- string
- Default:
- null
Explicitly set data source type (such as "json"). Please refer to the documentation of $.ig.DataSource and its type property.
Code Sample
// Initialization $(".selector").igMap({ dataSourceType: "array" }); // Get var dataSourceType = $(".selector").igMap("option", "dataSourceType"); // Set $(".selector").igMap("option", "dataSourceType", "array");
-
dataSourceUrl
- Type:
- string
- Default:
- null
Specifies a remote URL accepted by $.ig.DataSource in order to request data from it.
Code Sample
// Initialization $(".selector").igMap({ dataSourceUrl: "http://www.example.com" }); // Get var dataSourceUrl = $(".selector").igMap("option", "dataSourceUrl"); // Set $(".selector").igMap("option", "dataSourceUrl", "http://www.example.com");
-
defaultInteraction
- Type:
- enumeration
- Default:
- dragpan
Gets or sets the DefaultInteraction property. The default interaction state defines the map's response to mouse events.
Members
- none
- Type:string
- User gesture will not change the state of the map.
- dragZoom
- Type:string
- User gesture will start a drag rectangle to zoom the map.
- dragPan
- Type:string
- User gesture will start a pan action to move the map's window.
Code Sample
// Initialization $(".selector").igMap({ defaultInteraction: "dragZoom" }); // Get var defaultInteraction = $(".selector").igMap("option", "defaultInteraction"); // Set $(".selector").igMap("option", "defaultInteraction", "dragZoom");
-
diamondMarkerTemplate
- Type:
- object
- Default:
- null
Gets or sets the template to use for diamond markers on the map.
Defines the marker template used for
series with a marker type of diamond.
The provided object should have properties called render and optionally measure. See definition for option: legendItemBadgeTemplate.Code Sample
//Initialize $(".selector").igMap({ diamondMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
dragModifier
- Type:
- enumeration
- Default:
- none
Gets or sets the current Map's DragModifier property.
Members
- none
- Type:string
- No modifier key is set.
- alt
- Type:string
- The modifier is set to alt key.
- control
- Type:string
- The modifier is set to control key.
- shift
- Type:string
- The modifier is set to shift key.
Code Sample
// Initialization $(".selector").igMap({ dragModifier: "control" }); // Get var dragModifier = $(".selector").igMap("option", "dragModifier"); // Set $(".selector").igMap("option", "dragModifier", "control");
-
height
- Type:
- number
- Default:
- null
The height of the map. It can be set as a number in pixels, string (px) or percentage (%).
Code Sample
// Initialization $(".selector").igMap({ height: 250 }); // Get var height = $(".selector").igMap("option", "height"); // Set $(".selector").igMap("option", "height", 250);
-
hexagonMarkerTemplate
- Type:
- object
- Default:
- null
Gets or sets the template to use for hexagon markers on the map.
Defines the marker template used for
series with a marker type of hexagon.
The provided object should have properties called render and optionally measure. See definition for option: legendItemBadgeTemplate.Code Sample
//Initialize $(".selector").igMap({ hexagonMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
hexagramMarkerTemplate
- Type:
- object
- Default:
- null
Gets or sets the template to use for hexagram markers on the map.
Defines the marker template used for
series with a marker type of hexagram.
The provided object should have properties called render and optionally measure. See definition for option: legendItemBadgeTemplate.Code Sample
//Initialize $(".selector").igMap({ hexagramMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
overviewPlusDetailPaneBackgroundImageUri
- Type:
- string
- Default:
- null
The background image uri use in the overview detail pane.
Code Sample
// Initialization $(".selector").igMap({ overviewPlusDetailPaneBackgroundImageUri: "Content/img/World.png" }); // Get var OPDImageUri = $(".selector").igMap("option", "overviewPlusDetailPaneBackgroundImageUri"); // Set $(".selector").igMap("option", "overviewPlusDetailPaneBackgroundImageUri", "Content/img/World.png");
-
panModifier
- Type:
- enumeration
- Default:
- shift
Gets or sets the current Map's PanModifier property.
Members
- none
- Type:string
- No modifier key is set.
- alt
- Type:string
- The modifier is set to alt key.
- control
- Type:string
- The modifier is set to control key.
- shift
- Type:string
- The modifier is set to shift key.
Code Sample
// Initialization $(".selector").igMap({ panModifier: "control" }); // Get var panModifier = $(".selector").igMap("option", "panModifier"); // Set $(".selector").igMap("panModifier", "control");
-
pentagonMarkerTemplate
- Type:
- object
- Default:
- null
Gets or sets the template to use for pentagon markers on the map.
Defines the marker template used for
series with a marker type of pentagon.
The provided object should have properties called render and optionally measure. See definition for option: legendItemBadgeTemplate.Code Sample
//Initialize $(".selector").igMap({ pentagonMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
pentagramMarkerTemplate
- Type:
- object
- Default:
- null
Gets or sets the template to use for pentragram markers on the map.
Defines the marker template used for
series with a marker type of pentagram.
The provided object should have properties called render and optionally measure. See definition for option: legendItemBadgeTemplate.Code Sample
//Initialize $(".selector").igMap({ pentagramMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
plotAreaBackground
- Type:
- string
- Default:
- null
Gets or sets the brush used as the background for the current Map object's plot area.
Code Sample
//Initialize $(".selector").igMap({ plotAreaBackground: "grey" }); //Get var plotAreaBackground = $(".selector").igMap("option", "plotAreaBackground"); //Set var plotAreaBackground = $(".selector").igMap("option", "plotAreaBackground", "grey");
-
previewRect
- Type:
- object
- Default:
- null
Gets or sets the preview rectangle.
The preview rectangle may be set to Rect.Empty, in which case the visible preview
strokePath is hidden.
The provided object should have numeric properties called left, top, width and height.Code Sample
// Initialization $(".selector").igMap({ previewRect: { left: 0.3, top: 0.3, width: 0.5, height: 0.5 } }); // Get var previewRect = $(".selector").igMap("option", "previewRect"); // Set $(".selector").igMap("previewRect", { left: 0.3, top: 0.3, width: 0.5, height: 0.5 });
-
pyramidMarkerTemplate
- Type:
- object
- Default:
- null
Gets or sets the template to use for pyramid markers on the map.
Defines the marker template used for
series with a marker type of pyramid.
The provided object should have properties called render and optionally measure. See definition for option: legendItemBadgeTemplate.Code Sample
//Initialize $(".selector").igMap({ pyramidMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
responseDataKey
- Type:
- string
- Default:
- null
See $.ig.DataSource. Specifies the name of the property in which data records are held if the response is wrapped.
Code Sample
//Initialize $(".selector").igMap({ responseDataKey: "Records" }); //Get var responseDataKey = $(".selector").igMap("option", "responseDataKey"); //Set var responseDataKey = $(".selector").igMap("option", "responseDataKey", "Records");
-
series
- Type:
- array
- Default:
- []
- Elements Type:
- object
An array of series objects.
Code Sample
//Initialization var data = [ { Name: "London", Country: "England", Latitude: 51.50, Longitude: 0.12 }, { Name: "Berlin", Country: "Germany", Latitude: 52.50, Longitude: 13.33 } ]; $("#map").igMap({ series: [{ type: "geographicSymbol", name: "worldCities", dataSource: data, latitudeMemberPath: "Latitude", longitudeMemberPath: "Longitude" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0]; //Set $(".selector").igMap("option", "series", [{ type: "geographicSymbol", name: "worldCities", latitudeMemberPath: "Latitude", longitudeMemberPath: "Longitude" }] );
-
angleMemberPath
- Type:
- number
- Default:
- null
Gets or sets the x-radius of the ellipse that is used to round the corners of the column.
Code Sample
//Initialization $(".selector").igMap({ series: [{ angleMemberPath: "Index" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].angleMemberPath; //Set $(".selector").igMap("option", "series", [{ angleMemberPath: "Index" }]);
-
brush
- Type:
- string
- Default:
- null
Gets or sets the brush to use for the series.
Code Sample
//Initialization $("#map").igMap({ series: [{ brush: "blue" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].brush; //Set $(".selector").igMap("option", "series", [{ brush: "blue" }]);
-
clipSeriesToBounds
- Type:
- bool
- Default:
- null
Gets or sets whether to clip the series to the bounds.
Setting this to true can affect performance.Code Sample
//Initialization $("#map").igMap({ series: [{ clipSeriesToBounds: true }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].clipSeriesToBounds; //Set $(".selector").igMap("option", "series", [{ clipSeriesToBounds: true }]);
-
closeMemberPath
- Type:
- string
- Default:
- null
Gets or sets the close mapping property for the current series object.
Code Sample
//Initialization $("#map").igMap({ series: [{ closeMemberPath: "Close" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].closeMemberPath; //Set $(".selector").igMap("option", "series", [{ closeMemberPath: "Close" }]);
-
colorMemberPath
- Type:
- string
- Default:
- null
The name of the property on each data item containing a numeric value which can be converted to a color by the ColorScale.
Code Sample
//Initialization $("#map").igMap({ series: [{ colorMemberPath: "Temperature" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].colorMemberPath; //Set $(".selector").igMap("option", "series", [{ colorMemberPath: "Temperature" }]);
-
colorScale
- Type:
- object
- Default:
- null
The ColorScale used to resolve the color values of points in the series.
Code Sample
//Initialization $("#map").igMap({ series: [{ name: "sampleSeries", type: "geographicScatterArea", colorScale: { type: "customPalette", interpolationMode: "interpolateRGB", palette: [ "#3300CC", "#4775FF" ] } }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].colorScale; //Set $(".selector").igMap("option", "series", [{ name: "sampleSeries", colorScale: { type: "customPalette", interpolationMode: "interpolateRGB", palette: [ "#3300CC", "#4775FF" ] } }]);
-
databaseSource
- Type:
- string
- Default:
- null
String The database source URI.
Code Sample
//Initialization $("#map").igMap({ series: [{ name: "sampleSeries", type: "geographicShape", databaseSource: "ShapeData.dbf" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].databaseSource; //Set $(".selector").igMap("option", "series", [{ name: "sampleSeries", databaseSource: "ShapeData.dbf" }]);
-
dataSource
- Type:
- object
- Default:
- null
Can be any valid data source accepted by $.ig.DataSource, or an instance of an $.ig.DataSource itself.
Code Sample
//Initialization $("#map").igMap({ series: [{ dataSource: data }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].dataSource; //Set $(".selector").igMap("option", "series", [{ dataSource: data }]);
-
dataSourceType
- Type:
- string
- Default:
- null
Explicitly set data source type (such as "json"). Please refer to the documentation of $.ig.DataSource and its type property.
Code Sample
//Initialization $("#map").igMap({ series: [{ dataSourceType: "array" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].dataSourceType; //Set $(".selector").igMap("option", "series", [{ dataSourceType: "array" }]);
-
dataSourceUrl
- Type:
- string
- Default:
- null
Specifies a remote URL accepted by $.ig.DataSource in order to request data from it.
Code Sample
//Initialization $("#map").igMap({ series: [{ dataSourceUrl: "http://www.example.com/map-data" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].dataSourceUrl; //Set $(".selector").igMap("option", "series", [{ dataSourceUrl: "http://www.example.com/map-data" }]);
-
discreteLegendItemTemplate
- Type:
- object
- Default:
- null
Gets or sets the DiscreteLegendItemTemplate property.
The legend item control content is created according to the DiscreteLegendItemTemplate on-demand by
the series object itself.
The provided object should have properties called render and optionally measure. See definition for option: legendItemBadgeTemplate.Code Sample
//Initialization $("#map").igMap({ series: [{ discreteLegendItemTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } }] });
-
fillMemberPath
- Type:
- string
- Default:
- null
The name of the property on data source items which contains a numeric value to convert to a Brush using the FillScale.
Code Sample
//Initialization $(".selector").igMap({ series: [{ name: "sampleSeries", type: "geographicContourLine", fillMemberPath: "Temperature" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].fillMemberPath; //Set $(".selector").igMap("option", "series", [{ name: "series1", fillMemberPath: "Temperature" }]);
-
fillScale
- Type:
- object
- Default:
- null
The ValueBrushScale to use when determining Brushes for each Shape, based on the values found in FillMemberPath.
Code Sample
//Initialization $(".selector").igMap({ series: [{ name: "sampleSeries", type: "geographicContourLine", fillScale: { type: "value", brushes: ["#3300CC", "#4775FF", "#0099CC", "#00CC99", "#33CC00", "#99CC00", "#CC9900", "#FFC20A", "#CC3300"] } }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].fillScale; //Set $(".selector").igMap("option", "series", [{ name: "series1", fillScale: { type: "value", brushes: ["#3300CC", "#4775FF", "#0099CC", "#00CC99", "#33CC00", "#99CC00", "#CC9900", "#FFC20A", "#CC3300"] } }]);
-
heatMaximum
- Type:
- number
- Default:
- 50
Gets or sets the value that maps to the maximum heat color.
Code Sample
//Initialization $("#map").igMap({ series: [{ heatMaximum: 100 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].heatMaximum; //Set $(".selector").igMap("option", "series", [{ heatMaximum: 100 }]);
-
heatMinimum
- Type:
- number
- Default:
- 0
Gets or sets the density value that maps to the minimum heat color.
Code Sample
//Initialization $("#map").igMap({ series: [{ heatMinimum: 5 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].heatMinimum; //Set $(".selector").igMap("option", "series", [{ heatMinimum: 5 }]);
-
highMemberPath
- Type:
- string
- Default:
- null
Gets or sets the value mapping property for the current series object.
Code Sample
//Initialization $("#map").igMap({ series: [{ highMemberPath: "High" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].highMemberPath; //Set $(".selector").igMap("option", "series", [{ highMemberPath: "High" }]);
-
ignoreFirst
- Type:
- number
- Default:
- 0
Gets or sets the number of values to hide at the beginning of the indicator.
Code Sample
//Initialization $("#map").igMap({ series: [{ ignoreFirst: 5 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].ignoreFirst; //Set $(".selector").igMap("option", "series", [{ ignoreFirst: 5 }]);
-
labelMemberPath
- Type:
- string
- Default:
- null
Gets or sets the Label mapping property for the current series object.
Code Sample
//Initialization $("#map").igMap({ series: [{ labelMemberPath: "Name" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].labelMemberPath; //Set $(".selector").igMap("option", "series", [{ labelMemberPath: "Name" }]);
-
latitudeMemberPath
- Type:
- string
- Default:
- null
The name of the property of data source items which contains the latitude coordinate of the symbol.
Code Sample
//Initialization $("#map").igMap({ series: [{ latitudeMemberPath: "Latitude" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].latitudeMemberPath; //Set $(".selector").igMap("option", "series", [{ latitudeMemberPath: "Latitude" }]);
-
legendItemBadgeTemplate
- Type:
- object
- Default:
- null
Gets or sets the LegendItemBadgeTemplate property.
The legend item badge is created according to the LegendItemBadgeTemplate on-demand by
the series object itself.
The provided object should have properties called render and optionally measure.
These are functions which will be called that will be called to handle the user specified custom rendering.
measure will be passed an object that looks like this:
{
context: [either a DOM element or a CanvasContext2D depending on the particular template scenario],
width: [if value is present, specifies the available width, user may set to desired width for content],
height: [if value is present, specifies the available height, user may set to desired height for content],
isConstant: [user should set to true if desired with and height will always be the same for this template],
data: [if present, represents the contextual data for this template]
}
render will be passed an object that looks like this:
{
context: [either a DOM element or a CanvasContext2D depending on the particular template scenario],
xPosition: [if present, specifies the x position at which to render the content],
yPosition: [if present, specifies the y position at which to render the content],
availableWidth: [if present, specifies the available width in which to render the content],
availableHeight: [if present, specifies the available height in which to render the content],
data: [if present, specifies the data that is in context for this content],
isHitTestRender: [if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used]
}.Code Sample
//Initialization $("#map").igMap({ series: [{ legendItemBadgeTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } }] });
-
legendItemTemplate
- Type:
- object
- Default:
- null
Gets or sets the LegendItemTemplate property.
The legend item control content is created according to the LegendItemTemplate on-demand by
the series object itself.
The provided object should have properties called render and optionally measure. See definition for option: legendItemBadgeTemplate.Code Sample
//Initialization $("#map").igMap({ series: [{ legendItemTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } }] });
-
longitudeMemberPath
- Type:
- string
- Default:
- null
The name of the property of data source items which contains the longitude coordinate of the symbol.
Code Sample
//Initialization $("#map").igMap({ series: [{ longitudeMemberPath: "Longitude" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].longitudeMemberPath; //Set $(".selector").igMap("option", "series", [{ longitudeMemberPath: "Longitude" }]);
-
longPeriod
- Type:
- number
- Default:
- 0
Gets or sets the short moving average period for the current AbsoluteVolumeOscillatorIndicator object.
The typical, and initial, value for long AVO periods is 30.Code Sample
//Initialization $("#map").igMap({ series: [{ longPeriod: 50 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].longPeriod; //Set $(".selector").igMap("option", "series", [{ longPeriod: 50 }]);
-
lowMemberPath
- Type:
- string
- Default:
- null
Gets or sets the value mapping property for the current series object.
Code Sample
//Initialization $("#map").igMap({ series: [{ lowMemberPath: "Low" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].lowMemberPath; //Set $(".selector").igMap("option", "series", [{ lowMemberPath: "Low" }]);
-
markerBrush
- Type:
- string
- Default:
- null
Gets or sets the brush that specifies how the current series object's marker interiors are painted.
Code Sample
//Initialization $("#map").igMap({ series: [{ markerBrush: "blue" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].markerBrush; //Set $(".selector").igMap("option", "series", [{ markerBrush: "blue" }]);
-
markerCollisionAvoidance
- Type:
- enumeration
- Default:
- none
Gets or sets the MarkerCollisionAvoidance .
Members
- none
- Type:string
- No collision avoidance is attempted.
- omit
- Type:string
- Markers that collide will be omitted.
- fade
- Type:string
- Markers that collide will be faded in opacity.
- omitAndShift
- Type:string
- Markers that collide may be shifted or omitted.
Code Sample
//Initialization $("#map").igMap({ series: [{ markerCollisionAvoidance: "fadeAndShift" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].markerCollisionAvoidance; //Set $(".selector").igMap("option", "series", [{ markerCollisionAvoidance: "fadeAndShift" }]);
-
markerOutline
- Type:
- string
- Default:
- null
Gets or sets the brush that specifies how the current series object's marker outlines are painted.
Code Sample
//Initialization $("#map").igMap({ series: [{ markerOutline: "black" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].markerOutline; //Set $(".selector").igMap("option", "series", [{ markerOutline: "black" }]);
-
markerTemplate
- Type:
- object
- Default:
- null
Gets or sets the MarkerTemplate for the current series object.
The provided object should have properties called render and optionally measure.
These are functions which will be called that will be called to handle the user specified custom rendering.
measure will be passed an object that looks like this:
{
context: [either a DOM element or a CanvasContext2D depending on the particular template scenario],
width: [if value is present, specifies the available width, user may set to desired width for content],
height: [if value is present, specifies the available height, user may set to desired height for content],
isConstant: [user should set to true if desired with and height will always be the same for this template],
data: [if present, represents the contextual data for this template]
}
render will be passed an object that looks like this:
{
context: [either a DOM element or a CanvasContext2D depending on the particular template scenario],
xPosition: [if present, specifies the x position at which to render the content],
yPosition: [if present, specifies the y position at which to render the content],
availableWidth: [if present, specifies the available width in which to render the content],
availableHeight: [if present, specifies the available height in which to render the content],
data: [if present, specifies the data that is in context for this content],
isHitTestRender: [if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used]
}.Code Sample
//Initialization $("#map").igMap({ series: [{ markerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } }] });
-
markerType
- Type:
- enumeration
- Default:
- none
Gets or sets the marker type for the current series object. If the MarkerTemplate property is set, the setting of the MarkerType property will be ignored.
Members
- unset
- Type:string
- .
- none
- Type:string
- .
- automatic
- Type:string
- .
- circle
- Type:string
- .
- triangle
- Type:string
- .
- pyramid
- Type:string
- .
- square
- Type:string
- .
- diamond
- Type:string
- .
- pentagon
- Type:string
- .
- hexagon
- Type:string
- .
- tetragram
- Type:string
- .
- pentagram
- Type:string
- .
- hexagram
- Type:string
Code Sample
//Initialization $("#map").igMap({ series: [{ markerType: "square" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].markerType; //Set $(".selector").igMap("option", "series", [{ markerType: "square" }]);
-
maximumMarkers
- Type:
- number
- Default:
- 400
Gets or sets the maximum number of markerItems displayed by the current series.
If more than the specified number of markerItems are visible, the series will automatically
choose a representative set.Code Sample
//Initialization $("#map").igMap({ series: [{ maximumMarkers: 150 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].maximumMarkers; //Set $(".selector").igMap("option", "series", [{ maximumMarkers: 150 }]);
-
mouseOverEnabled
- Type:
- bool
- Default:
- false
Gets or sets the whether the map reacts to mouse move events.
Code Sample
//Initialization $("#map").igMap({ series: [{ mouseOverEnabled: true }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].mouseOverEnabled; //Set $(".selector").igMap("option", "series", [{ mouseOverEnabled: true }]);
-
name
- Type:
- string
- Default:
- null
The unique identifier of the series.
Code Sample
//Initialization $("#map").igMap({ series: [{ name: "seriesName" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].name; //Set $(".selector").igMap("option", "series", [{ name: "seriesName" }]);
-
negativeBrush
- Type:
- string
- Default:
- null
Gets or sets the brush to use for negative portions of the series.
Code Sample
//Initialization $("#map").igMap({ series: [{ negativeBrush: "red" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].negativeBrush; //Set $(".selector").igMap("option", "series", [{ negativeBrush: "red" }]);
-
openMemberPath
- Type:
- string
- Default:
- null
Gets or sets the value mapping property for the current series object.
Code Sample
//Initialization $("#map").igMap({ series: [{ openMemberPath: "Open" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].openMemberPath; //Set $(".selector").igMap("option", "series", [{ openMemberPath: "Open" }]);
-
outline
- Type:
- string
- Default:
- null
Gets or sets the brush to use for the outline of the series.
Some series types, such as LineSeries, do not display outlines.Code Sample
//Initialization $("#map").igMap({ series: [{ outline: "black" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].outline; //Set $(".selector").igMap("option", "series", [{ outline: "black" }]);
-
period
- Type:
- number
- Default:
- 0
Gets or sets the moving average period for the current AverageDirectionalIndexIndicator object.
The typical, and initial, value for AverageDirectionalIndexIndicator periods is 14.Code Sample
//Initialization $("#map").igMap({ series: [{ period: 45 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].period; //Set $(".selector").igMap("option", "series", [{ period: 45 }]);
-
progressiveLoad
- Type:
- bool
- Default:
- true
Gets or sets the whether to progressively load the data into the map.
Code Sample
//Initialization $("#map").igMap({ series: [{ progressiveLoad: false }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].progressiveLoad; //Set $(".selector").igMap("option", "series", [{ progressiveLoad: false }]);
-
radiusMemberPath
- Type:
- string
- Default:
- null
Gets or sets the radius mapping property for the current series object.
Code Sample
//Initialization $("#map").igMap({ series: [{ radiusMemberPath: "Sales" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].radiusMemberPath; //Set $(".selector").igMap("option", "series", [{ radiusMemberPath: "Sales" }]);
-
radiusScale
- Type:
- object
- Default:
- null
Gets or sets the radius size scale for the bubbles.
Code Sample
//Initialization $("#map").igMap({ series: [{ radiusScale: 0.8 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].radiusScale; //Set $(".selector").igMap("option", "series", [{ radiusScale: 0.8 }]);
-
remove
- Type:
- bool
- Default:
- false
Set to true in order to have an existing series removed from the map, by name.
Code Sample
//Set $(".selector").igMap("option", "series", [{ name: "series1", remove: true }]);
-
resolution
- Type:
- number
- Default:
- 1
Gets or sets the current series object's rendering resolution.
Code Sample
//Initialization $("#map").igMap({ series: [{ resolution: 300 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].resolution; //Set $(".selector").igMap("option", "series", [{ resolution: true }]);
-
responseDataKey
- Type:
- string
- Default:
- null
See $.ig.DataSource. Specifies the name of the property in which data records are held if the response is wrapped.
Code Sample
//Initialization $("#map").igMap({ series: [{ responseDataKey: "Results" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].responseDataKey; //Set $(".selector").igMap("option", "series", [{ responseDataKey: "Results" }]);
-
shapeDataSource
- Type:
- string
- Default:
- null
The triangulated file source URI or an instance of $.ig.ShapeDataSource.
Code Sample
//Initialization $("#map").igMap({ series: [{ shapeDataSource: "ShapeData.shp" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].shapeDataSource; //Set $(".selector").igMap("option", "series", [{ shapeDataSource: "ShapeData.shp" }]);
-
shapeFilterResolution
- Type:
- number
- Default:
- 2
Gets or sets the resolution at which to filter out shapes in the series.
For example, if the shapeFilterResolution is set to 3, then elements with a bounding rectangle smaller than 3 X 3 pixels will be filtered out.Code Sample
//Initialization $("#map").igMap({ series: [{ shapeFilterResolution: 50 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].shapeFilterResolution; //Set $(".selector").igMap("option", "series", [{ shapeFilterResolution: 50 }]);
-
shapeMemberPath
- Type:
- string
- Default:
- null
The name of the property on data source items which, for each shape, contains a list of points to be converted to a polygon.
To be consistent with the Shapefile technical description, it is expected that each list of points is defined as an IEnumerable of IEnumerable of Point, or in other words, a list of lists of points.Code Sample
//Initialization $("#map").igMap({ series: [{ shapeMemberPath: "points" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].shapeMemberPath; //Set $(".selector").igMap("option", "series", [{ shapeMemberPath: "points" }]);
-
shapeStyle
- Type:
- object
- Default:
- null
The default style to apply to all Shapes in the series.
Code Sample
//Initialization $("#map").igMap({ series: [{ shapeStyle: { fill: "blue", stroke: "black" } }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].shapeStyle; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", shapeStyle: { fill: "blue", stroke: "black" } }]);
-
shapeStyleSelector
- Type:
- object
- Default:
- null
The StyleSelector which is used to select a style for each Shape.
Code Sample
//Initialization $("#map").igMap({ series: [{ shapeStyleSelector: { selectStyle = function (shapeData, o) { var shapeID = shapeData.fields.item("ID"); return { fill: "blue", stroke: "black" }; } } }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].shapeStyleSelector; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", shapeStyleSelector: { selectStyle = function (shapeData, o) { var shapeID = shapeData.fields.item("ID"); return { fill: "blue", stroke: "black" }; } } }]);
-
shortPeriod
- Type:
- number
- Default:
- 0
Gets or sets the short moving average period for the current AbsoluteVolumeOscillatorIndicator object.
The typical, and initial, value for short AVO periods is 10.Code Sample
//Initialization $("#map").igMap({ series: [{ shortPeriod: 15 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].shortPeriod; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", shortPeriod: 15 }]);
-
showTooltip
- Type:
- bool
- Default:
- false
Whether the map should render a tooltip.
Code Sample
//Initialization $("#map").igMap({ series: [{ showTooltip: true }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].showTooltip; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", showTooltip: true }]);
-
splineType
- Type:
- enumeration
- Default:
- natural
Gets or sets the type of spline to be rendered.
Members
- natural
- Type:string
- Calculates the spline using a natural spline calculation formula. .
- clamped
- Type:string
- Calculated the spline using a clamped spline calculation formula.
Code Sample
//Initialization $("#map").igMap({ series: [{ splineType: "clamped" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].splineType; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", splineType: "clamped" }]);
-
stiffness
- Type:
- number
- Default:
- 0.5
Gets or sets the Stiffness property.
Code Sample
//Initialization $("#map").igMap({ series: [{ stiffness: 0.8 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].stiffness; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", stiffness: 0.8 }]);
-
thickness
- Type:
- number
- Default:
- 0
Gets or sets the width of the current series object's line thickness.
Code Sample
//Initialization $("#map").igMap({ series: [{ thickness: 2 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].thickness; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", thickness: 2 }]);
-
title
- Type:
- string
- Default:
- null
Gets or sets the Title property.
The legend item control is created according to the Title on-demand by
the series object itself.Code Sample
//Initialization $("#map").igMap({ series: [{ title: "Sales" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].title; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", title: "Sales" }]);
-
transitionDuration
- Type:
- number
- Default:
- 0
Gets or sets the duration of the current series's morph.
Code Sample
//Initialization $("#map").igMap({ series: [{ transitionDuration: 500 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].transitionDuration; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", transitionDuration: 500 }]);
-
trendLineBrush
- Type:
- string
- Default:
- null
Gets or sets the brush to use to draw the trend line.
Code Sample
//Initialization $("#map").igMap({ series: [{ trendLineBrush: "green" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].trendLineBrush; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", trendLineBrush: "green" }]);
-
trendLinePeriod
- Type:
- number
- Default:
- 7
Gets or sets the moving average period for the current scatter series object.
The typical, and initial, value for trend line period is 7.Code Sample
//Initialization $("#map").igMap({ series: [{ trendLinePeriod: 20 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].trendLinePeriod; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", trendLinePeriod: 20 }]);
-
trendLineThickness
- Type:
- number
- Default:
- 1.5
Gets or sets the thickness of the current scatter series object's trend line.
Code Sample
//Initialization $("#map").igMap({ series: [{ trendLineThickness: 3 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].trendLineThickness; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", trendLineThickness: 3 }]);
-
trendLineType
- Type:
- enumeration
- Default:
- none
Gets or sets the trend type for the current scatter series.
Members
- none
- Type:string
- No trendline should display. .
- linearFit
- Type:string
- Linear fit. .
- quadraticFit
- Type:string
- Quadratic polynomial fit. .
- cubicFit
- Type:string
- Cubic polynomial fit. .
- quarticFit
- Type:string
- Quartic polynomial fit. .
- quinticFit
- Type:string
- Quintic polynomial fit. .
- logarithmicFit
- Type:string
- Logarithmic fit. .
- exponentialFit
- Type:string
- Exponential fit. .
- powerLawFit
- Type:string
- Powerlaw fit. .
- simpleAverage
- Type:string
- Simple moving average. .
- exponentialAverage
- Type:string
- Exponential moving average. .
- modifiedAverage
- Type:string
- Modified moving average. .
- cumulativeAverage
- Type:string
- Cumulative moving average. .
- weightedAverage
- Type:string
- Weighted moving average.
Code Sample
//Initialization $("#map").igMap({ series: [{ trendLineType: "logarithmicFit" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].trendLineType; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", trendLineType: "logarithmicFit" }]);
-
trendLineZIndex
- Type:
- number
- Default:
- 1001
Gets or sets the Z-Index of the trend line. Values greater than 1000 will result in the trend line being rendered in front of the series data.
Code Sample
//Initialization $("#map").igMap({ series: [{ trendLineZIndex: 500 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].trendLineZIndex; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", trendLineZIndex: 500 }]);
-
trianglesSource
- Type:
- object
- Default:
- null
The source of triangulation data.
This property is optional. If it is left as null, the triangulation will be created based on the items in the data source. Triangulation is a demanding operation, so the runtime performance will be better when specifying a TriangulationSource, especially when a large number of data items are present.Code Sample
var data = [ { longitude: 0, latitude: 0, value: 1 }, { longitude: 50, latitude: 0, value: 2 }, { longitude: 50, latitude: 50, value: 3 }, { longitude: 0, latitude: 50, value: 1 } ]; // Initialization $("#map").igMap({ series: [{ name: "series1", type: "geographicScatterArea", latitudeMemberPath: "latitude", longitudeMemberPath: "longitude", colorMemberPath: "value", colorScale: { minimumValue: 1, interpolationMode: "select", palette: ["darkgreen", "green", 'limegreen', 'lightgreen'] }, trianglesSource: data }] }); // Get var series = $(".selector").igMap("option", "series"); series[0].trianglesSource; // Set $(".selector").igMap("option", "series", [{ name: "series1", type: "geographicScatterArea", latitudeMemberPath: "latitude", longitudeMemberPath: "longitude", colorMemberPath: "value", colorScale: { minimumValue: 1, interpolationMode: "select", palette: ["darkgreen", "green", 'limegreen', 'lightgreen'] }, trianglesSource: data }]);
-
triangleVertexMemberPath1
- Type:
- string
- Default:
- null
The name of the property of the TrianglesSource items which, for each triangle, contains the index of the first vertex point in the data source.
Code Sample
//Initialization $("#map").igMap({ series: [{ triangleVertexMemberPath1: "v1" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].triangleVertexMemberPath1; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", triangleVertexMemberPath1: "v1" }]);
-
triangleVertexMemberPath2
- Type:
- string
- Default:
- null
The name of the property of the TrianglesSource items which, for each triangle, contains the index of the second vertex point in the data source.
Code Sample
//Initialization $("#map").igMap({ series: [{ triangleVertexMemberPath2: "v2" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].triangleVertexMemberPath2; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", triangleVertexMemberPath2: "v2" }]);
-
triangleVertexMemberPath3
- Type:
- string
- Default:
- null
The name of the property of the TrianglesSource items which, for each triangle, contains the index of the third vertex point in the data source.
Code Sample
//Initialization $("#map").igMap({ series: [{ triangleVertexMemberPath3: "v3" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].triangleVertexMemberPath3; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", triangleVertexMemberPath3: "v3" }]);
-
triangulationDataSource
- Type:
- string
- Default:
- null
The triangulated file source URI or an instance of $.ig.TriangulationDataSource.
Code Sample
//Initialization $("#map").igMap({ series: [{ triangulationDataSource: "Triangulation.itf" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].triangulationDataSource; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", triangulationDataSource: "Triangulation.itf" }]);
-
type
- Type:
- enumeration
- Default:
- null
Type of the series.
Members
- geographicSymbolSeries
- Type:string
- Specify the series as geographic Symbol Series series.
- geographicPolyLine
- Type:string
- Specify the series as geographic Poly Line series.
- geographicScatterArea
- Type:string
- Specify the series as geographic Scatter Area series.
- geographicShape
- Type:string
- Specify the series as geographic Shape series.
- geographicContourLine
- Type:string
- Specify the series as geographic Contour Line series.
- geographicHighDensityScatter
- Type:string
- Specify the series as geographic High Density Scatter series.
- geographicProportionalSize
- Type:string
- Specify the series as geographic Proportional Size series.
Code Sample
//Initialization $("#map").igMap({ series: [{ type: "geographicPolyLine" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].type; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", type: "geographicPolyLine" }]);
-
unknownValuePlotting
- Type:
- enumeration
- Default:
- dontplot
Determines how unknown values will be plotted on the map. Null and Double.NaN are two examples of unknown values.
Members
- linearInterpolate
- Type:string
- Plot the unknown value as the midpoint between surrounding known values using linear interpolation.
- dontPlot
- Type:string
- Do not plot the unknown value on the map.
Code Sample
//Initialization $("#map").igMap({ series: [{ unknownValuePlotting: "linearInterpolate" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].unknownValuePlotting; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", unknownValuePlotting: "linearInterpolate" }]);
-
useBruteForce
- Type:
- bool
- Default:
- false
Gets or sets the whether to use use brute force mode.
Code Sample
//Initialization $("#map").igMap({ series: [{ useBruteForce: true }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].useBruteForce; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", useBruteForce: true }]);
-
useCartesianInterpolation
- Type:
- bool
- Default:
- true
Gets or sets whether Cartesian Interpolation should be used rather than Archimedian
spiral based interpolation.Code Sample
//Initialization $("#map").igMap({ series: [{ useCartesianInterpolation: false }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].useCartesianInterpolation; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", useCartesianInterpolation: false }]);
-
useSquareCutoffStyle
- Type:
- bool
- Default:
- false
Gets or sets the whether to use squares when halting a render traversal rather than the shape of the coalesced area.
Code Sample
//Initialization $("#map").igMap({ series: [{ useSquareCutoffStyle: true }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].useSquareCutoffStyle; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", useSquareCutoffStyle: true }]);
-
valueMemberPath
- Type:
- string
- Default:
- null
Gets or sets the item path that provides the values for the current series.
Code Sample
//Initialization $("#map").igMap({ series: [{ valueMemberPath: "Population" }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].valueMemberPath; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", valueMemberPath: "Population" }]);
-
valueResolver
- Type:
- object
- Default:
- null
Gets or sets the ContourValueResolver used to determine the numeric values of contours.
Code Sample
//Initialization $("#map").igMap({ series: { valueResolver: { type: "linear", valueCount: 300 } } }); //Get var series = $(".selector").igMap("option", "series"); series.valueResolver; //Set $(".selector").igMap("option", "series", { valueResolver: { type: "linear", valueCount: 300 } });
-
visibleFromScale
- Type:
- number
- Default:
- 0
The minimum scale at which this series becomes visible.
The default value for this property is 1.0, which means the series will always be visible. At a VisibleFromScale setting of 0.0, the series will never be visible. At a VisibleFromScale setting of 0.5, the series will be visible as long as the map is zoomed in to at least 200%.Code Sample
//Initialization $("#map").igMap({ series: [{ visibleFromScale: 0.5 }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].visibleFromScale; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", visibleFromScale: 0.5 }]);
-
volumeMemberPath
- Type:
- string
- Default:
- null
Gets or sets the volume mapping property for the current series object.
Code Sample
//Initialization $("#map").igMap({ series: [{ volumeMemberPath: Sales }] }); //Get var series = $(".selector").igMap("option", "series"); series[0].volumeMemberPath; //Set $(".selector").igMap("option", "series", [{ name: "seriesName", volumeMemberPath: Sales }]);
-
squareMarkerTemplate
- Type:
- object
- Default:
- null
Gets or sets the template to use for square markers on the map.
Defines the marker template used for
series with a marker type of square.
The provided object should have properties called render and optionally measure. See definition for option: legendItemBadgeTemplate.Code Sample
//Initialize $(".selector").igMap({ squareMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
tetragramMarkerTemplate
- Type:
- object
- Default:
- null
Gets or sets the template to use for tetragram markers on the map.
Defines the marker template used for
series with a marker type of tetragram.
The provided object should have properties called render and optionally measure. See definition for option: legendItemBadgeTemplate.Code Sample
//Initialize $(".selector").igMap({ tetragramMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
theme
- Type:
- string
- Default:
- c
The swatch used to style this widget.
Code Sample
//Initialize $(".selector").igMap({ theme: "metro" }); //Get var theme = $(".selector").igMap("option", "theme");
-
triangleMarkerTemplate
- Type:
- object
- Default:
- null
Gets or sets the template to use for triangle markers on the map.
Defines the marker template used for
series with a marker type of triangle.
The provided object should have properties called render and optionally measure. See definition for option: legendItemBadgeTemplate.Code Sample
//Initialize $(".selector").igMap({ triangleMarkerTemplate: { measure: function(measureInfo) { measureInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario measureInfo.width; // if value is present, specifies the available width, user may set to desired width for content measureInfo.height; // if value is present, specifies the available height, user may set to desired height for content measureInfo.isConstant; // user should set to true if desired with and height will always be the same for this template measureInfo.data; // if present, represents the contextual data for this template] }, render: function (renderInfo) { renderInfo.context; // either a DOM element or a CanvasContext2D depending on the particular template scenario renderInfo.xPosition; // if present, specifies the x position at which to render the content renderInfo.yPosition; // if present, specifies the y position at which to render the content renderInfo.availableWidth; // if present, specifies the available width in which to render the content renderInfo.availableHeight; // if present, specifies the available height in which to render the content renderInfo.data; // if present, specifies the data that is in context for this content renderInfo.isHitTestRender; // if true, indicates that this is a special render pass for hit testing, in which case the brushes from the data should be used } } });
-
width
- Type:
- number
- Default:
- null
The width of the map. It can be set as a number in pixels, string (px) or percentage (%).
Code Sample
// Initialization $(".selector").igMap({ width: 250 }); // Get var width = $(".selector").igMap("option", "width"); // Set $(".selector").igMap("option", "width", 250);
-
windowPositionHorizontal
- Type:
- number
- Default:
- 0
A number between 0 and 1 determining the position of the horizontal scroll.
This property is effectively a shortcut to the X position of the WindowRect property.Code Sample
// Initialization $(".selector").igMap({ windowPositionHorizontal: 0.25 }); // Get var windowPositionHorizontal = $(".selector").igMap("option", "windowPositionHorizontal"); // Set $(".selector").igMap("windowPositionHorizontal", 0.25);
-
windowPositionVertical
- Type:
- number
- Default:
- 0
A number between 0 and 1 determining the position of the vertical scroll.
This property is effectively a shortcut to the Y position of the WindowRect property.Code Sample
// Initialization $(".selector").igMap({ windowPositionVertical: 0.25 }); // Get var windowPositionVertical = $(".selector").igMap("option", "windowPositionVertical"); // Set $(".selector").igMap("windowPositionVertical", 0.25);
-
windowRect
- Type:
- object
- Default:
- null
A rectangle representing the portion of the map currently in view.
A rectangle at X=0, Y=0 with a Height and Width of 1 implies the entire plotting area is in view. A Height and Width of .5 would imply that the view is halfway zoomed in.
The provided object should have numeric properties called left, top, width and height.Code Sample
// Initialization $(".selector").igMap({ windowRect: { left: 0, top: 0, width: 0.5, height: 0.5 } }); // Get var windowRect = $(".selector").igMap("option", "windowRect"); // Set $(".selector").igMap("windowRect", { left: 0, top: 0, width: 0.5, height: 0.5 });
-
windowRectMinWidth
- Type:
- number
- Default:
- 0
Sets or gets the minimum width that the window rect is allowed to reach before being clamped.
Decrease this value if you want to allow for further zooming into the viewer.
If this value is lowered too much it can cause graphical corruption due to floating point arithmetic inaccuracy.Code Sample
// Initialization $(".selector").igMap({ windowRectMinWidth: 0.5 }); // Get var windowRectMinWidth = $(".selector").igMap("option", "windowRectMinWidth"); // Set $(".selector").igMap("windowRectMinWidth", 0.5);
-
windowResponse
- Type:
- enumeration
- Default:
- null
The response to user panning and zooming: whether to update the view immediately while the user action is happening, or to defer the update to after the user action is complete. The user action will be an action such as a mouse drag which causes panning and/or zooming to occur.
Members
- deferred
- Type:string
- Defer the view update until after the user action is complete. .
- immediate
- Type:string
- Update the view immediately while the user action is happening.
Code Sample
// Initialization $(".selector").igMap({ windowResponse: "immediate" }); // Get var windowResponse = $(".selector").igMap("option", "windowResponse"); // Set $(".selector").igMap("windowResponse", "immediate");
-
windowScale
- Type:
- number
- Default:
- 1
Gets or sets the current Map's zoom scale.
-
zoomable
- Type:
- bool
- Default:
- false
Gets or sets the current Map's zoomability.
For more information on how to interact with the Ignite UI controls' events, refer to
Using Events in Ignite UI.
-
browserNotSupported
- Cancellable:
- false
Event fired when the control is displayed on a non HTML5 compliant browser.
Code Sample
// Delegate $(document).delegate(".selector", "igmapbrowsernotsupported", function (evt, ui) { }); // Initialize $(".selector").igMap({ browserNotSupported: function (evt, ui) { } });
-
gridAreaRectChanged
- Cancellable:
- false
Occurs just after the current Map's grid area rectangle is changed.
The grid area may change as the result of the Map being resized.
Function takes arguments evt and ui.
Use ui.map to get reference to map object.
Use ui.newHeight to get new height value.
Use ui.newLeft to get new left value.
Use ui.newTop to get new top value.
Use ui.newWidth to get new top value.
Use ui.oldHeight to get old height value.
Use ui.oldLeft to get old left value.
Use ui.oldTop to get old top value.
Use ui.oldWidth to get old top value.Code Sample
// Delegate $(document).delegate(".selector", "igmapgridarearectchanged", function (evt, ui) { // Get reference to map object. ui.map; // Get new height value. ui.newHeight; // Get new left value. ui.newLeft; // Get new top value. ui.newTop; // Get new top value. ui.newWidth; // Get old height value. ui.oldHeight; // Get old left value. ui.oldLeft; // Get old top value. ui.oldTop; // Get old top value. ui.oldWidth; }); // Initialize $(".selector").igMap({ gridAreaRectChanged: function (evt, ui) { } });
-
refreshCompleted
- Cancellable:
- false
Raised when the map's processing for an update has completed.
Function takes arguments evt and ui.
Use ui.map to get reference to map object.Code Sample
// Delegate $(document).delegate(".selector", "igmaprefreshcompleted", function (evt, ui) { // Get reference to map object. ui.map; }); // Initialize $(".selector").igMap({ refreshCompleted: function (evt, ui) { } });
-
seriesCursorMouseMove
- Cancellable:
- false
Occurs when the cursors are moved over a series in this map.
Function takes arguments evt and ui.
Use ui.item to get reference to current series item object.
Use ui.map to get reference to map object.
Use ui.series to get reference to current series object.
Use ui.actualItemBrush to get item brush.
Use ui.actualSeriesBrush to get series brush.
Use ui.positionX to get mouse X position.
Use ui.positionY to get mouse Y position.Code Sample
// Delegate $(document).delegate(".selector", "igmapseriescursormousemove", function (evt, ui) { // Get reference to current series item object. ui.item; // Get reference to chart object. ui.map; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; // Get mouse X position. ui.positionX; // Get mouse Y position. ui.positionY; }); // Initialize $(".selector").igMap({ seriesCursorMouseMove: function (evt, ui) { } });
-
seriesMouseEnter
- Cancellable:
- false
Occurs when the left mouse pointer enters an element of this map.
Function takes arguments evt and ui.
Use ui.item to get reference to current series item object.
Use ui.map to get reference to map object.
Use ui.series to get reference to current series object.
Use ui.actualItemBrush to get item brush.
Use ui.actualSeriesBrush to get series brush.
Use ui.positionX to get mouse X position.
Use ui.positionY to get mouse Y position.Code Sample
// Delegate $(document).delegate(".selector", "igmapseriesmouseenter", function (evt, ui) { // Get reference to current series item object. ui.item; // Get reference to map object. ui.map; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; // Get mouse X position. ui.positionX; // Get mouse Y position. ui.positionY; }); // Initialize $(".selector").igMap({ seriesMouseEnter: function (evt, ui) { } });
-
seriesMouseLeave
- Cancellable:
- false
Occurs when the left mouse pointer leaves an element of this map.
Function takes arguments evt and ui.
Use ui.item to get reference to current series item object.
Use ui.map to get reference to map object.
Use ui.series to get reference to current series object.
Use ui.actualItemBrush to get item brush.
Use ui.actualSeriesBrush to get series brush.
Use ui.positionX to get mouse X position.
Use ui.positionY to get mouse Y position.Code Sample
// Delegate $(document).delegate(".selector", "igmapseriesmouseleave", function (evt, ui) { // Get reference to current series item object. ui.item; // Get reference to map object. ui.map; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; // Get mouse X position. ui.positionX; // Get mouse Y position. ui.positionY; }); // Initialize $(".selector").igMap({ seriesMouseLeave: function (evt, ui) { } });
-
seriesMouseLeftButtonDown
- Cancellable:
- false
Occurs when the left mouse button is pressed while the mouse pointer is over an element of this map.
Function takes arguments evt and ui.
Use ui.item to get reference to current series item object.
Use ui.map to get reference to map object.
Use ui.series to get reference to current series object.
Use ui.actualItemBrush to get item brush.
Use ui.actualSeriesBrush to get series brush.
Use ui.positionX to get mouse X position.
Use ui.positionY to get mouse Y position.Code Sample
// Delegate $(document).delegate(".selector", "igmapseriesmouseleftbuttondown", function (evt, ui) { // Get reference to current series item object. ui.item; // Get reference to map object. ui.map; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; // Get mouse X position. ui.positionX; // Get mouse Y position. ui.positionY; }); // Initialize $(".selector").igMap({ seriesMouseLeftButtonDown: function (evt, ui) { } });
-
seriesMouseLeftButtonUp
- Cancellable:
- false
Occurs when the left mouse button is released while the mouse pointer is over an element of this map.
Function takes arguments evt and ui.
Use ui.item to get reference to current series item object.
Use ui.map to get reference to map object.
Use ui.series to get reference to current series object.
Use ui.actualItemBrush to get item brush.
Use ui.actualSeriesBrush to get series brush.
Use ui.positionX to get mouse X position.
Use ui.positionY to get mouse Y position.Code Sample
// Delegate $(document).delegate(".selector", "igmapseriesmouseleftbuttonup", function (evt, ui) { // Get reference to current series item object. ui.item; // Get reference to map object. ui.map; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; // Get mouse X position. ui.positionX; // Get mouse Y position. ui.positionY; }); // Initialize $(".selector").igMap({ seriesMouseLeftButtonUp: function (evt, ui) { } });
-
seriesMouseMove
- Cancellable:
- false
Occurs when the left mouse pointer moves while over an element of this map.
Function takes arguments evt and ui.
Use ui.item to get reference to current series item object.
Use ui.map to get reference to map object.
Use ui.series to get reference to current series object.
Use ui.actualItemBrush to get item brush.
Use ui.actualSeriesBrush to get series brush.
Use ui.positionX to get mouse X position.
Use ui.positionY to get mouse Y position.Code Sample
// Delegate $(document).delegate(".selector", "igmapseriesmousemove", function (evt, ui) { // Get reference to current series item object. ui.item; // Get reference to map object. ui.map; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; // Get mouse X position. ui.positionX; // Get mouse Y position. ui.positionY; }); // Initialize $(".selector").igMap({ seriesMouseMove: function (evt, ui) { } });
-
tooltipHidden
- Cancellable:
- false
Event fired after a tooltip is hidden
Function takes arguments evt and ui.
Use ui.element to get reference to tooltip DOM element.
Use ui.item to get reference to current series item object.
Use ui.map to get reference to map object.
Use ui.series to get reference to current series object.
Use ui.actualItemBrush to get item brush.
Use ui.actualSeriesBrush to get series brush.Code Sample
// Delegate $(document).delegate(".selector", "igmaptooltiphidden", function (evt, ui) { // Get reference to tooltip DOM element. ui.element; // Get reference to current series item object. ui.item; // Get reference to map object. ui.map; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; }); // Initialize $(".selector").igMap({ tooltipHidden: function (evt, ui) { } });
-
tooltipHiding
- Cancellable:
- true
Event fired when the mouse has left a series and the tooltip is about to hide
Function takes arguments evt and ui.
Use ui.element to get reference to tooltip DOM element.
Use ui.item to get reference to current series item object.
Use ui.map to get reference to map object.
Use ui.series to get reference to current series object.
Use ui.actualItemBrush to get item brush.
Use ui.actualSeriesBrush to get series brush.Code Sample
// Delegate $(document).delegate(".selector", "igmaptooltiphiding", function (evt, ui) { // Get reference to tooltip DOM element. ui.element; // Get reference to current series item object. ui.item; // Get reference to map object. ui.map; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; }); // Initialize $(".selector").igMap({ tooltipHiding: function (evt, ui) { } });
-
tooltipShowing
- Cancellable:
- true
Event fired when the mouse has hovered on a series and the tooltip is about to show
Function takes arguments evt and ui.
Use ui.element to get reference to tooltip DOM element.
Use ui.item to get reference to current series item object.
Use ui.map to get reference to map object.
Use ui.series to get reference to current series object.
Use ui.actualItemBrush to get item brush.
Use ui.actualSeriesBrush to get series brush.Code Sample
// Delegate $(document).delegate(".selector", "igmaptooltipshowing", function (evt, ui) { // Get reference to tooltip DOM element. ui.element; // Get reference to current series item object. ui.item; // Get reference to chart object. ui.map; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; }); // Initialize $(".selector").igMap({ tooltipShowing: function (evt, ui) { } });
-
tooltipShown
- Cancellable:
- false
Event fired after a tooltip is shown
Function takes arguments evt and ui.
Use ui.element to get reference to tooltip DOM element.
Use ui.item to get reference to current series item object.
Use ui.map to get reference to map object.
Use ui.series to get reference to current series object.
Use ui.actualItemBrush to get item brush.
Use ui.actualSeriesBrush to get series brush.Code Sample
// Delegate $(document).delegate(".selector", "igmaptooltipshown", function (evt, ui) { // Get reference to tooltip DOM element. ui.element; // Get reference to current series item object. ui.item; // Get reference to map object. ui.map; // Get reference to current series object. ui.series; // Get item brush. ui.actualItemBrush; // Get series brush. ui.actualSeriesBrush; }); // Initialize $(".selector").igMap({ tooltipShown: function (evt, ui) { } });
-
triangulationStatusChanged
- Cancellable:
- false
Event fired when the status of an ongoing Triangulation has changed.
Function takes arguments evt and ui.
Use ui.map to get reference to map object.
Use ui.series to get reference to current series object.
Use ui.currentStatus to get current status.Code Sample
// Delegate $(document).delegate(".selector", "igmaptriangulationstatuschanged", function (evt, ui) { // Get reference to chart object. ui.map; // Get the current series object. ui.series; // Get the current triangulation status. ui.currentStatus; }); // Initialize $(".selector").igMap({ triangulationStatusChanged: function (evt, ui) { } });
-
windowRectChanged
- Cancellable:
- false
Occurs just after the current Map's window rectangle is changed.
Function takes arguments evt and ui.
Use ui.map to get reference to map object.
Use ui.newHeight to get new height value.
Use ui.newLeft to get new left value.
Use ui.newTop to get new top value.
Use ui.newWidth to get new top value.
Use ui.oldHeight to get old height value.
Use ui.oldLeft to get old left value.
Use ui.oldTop to get old top value.
Use ui.oldWidth to get old top value.Code Sample
// Delegate $(document).delegate(".selector", "igmapwindowrectchanged", function (evt, ui) { // Get reference to chart object. ui.map; // Get new height value. ui.newHeight; // Get new left value. ui.newLeft; // Get new top value. ui.newTop; // Get new top value. ui.newWidth; // Get old height value. ui.oldHeight; // Get old left value. ui.oldLeft; // Get old top value. ui.oldTop; // Get old top value. ui.oldWidth; }); // Initialize $(".selector").igMap({ windowRectChanged: function (evt, ui) { } });
-
addItem
- .igMap( "addItem", item:object, targetName:string );
Adds a new item to the data source and notifies the map.
- item
- Type:object
- The item that we want to add to the data source. .
- targetName
- Type:string
- The name of the series bound to the data source.
Code Sample
$(".selector").igMap("addItem", {"Latitude": 42.2, "Longitude": 22.5 }, "series1" );
-
destroy
- .igMap( "destroy" );
Destroys the widget.
Code Sample
$(".selector").igMap("destroy");
-
exportImage
- .igMap( "exportImage", [width:object], [height:object] );
- Return Type:
- object
- Return Type Description:
- Returns a IMG DOM element.
Exports the map to a PNG image.
- width
- Type:object
- Optional
- The width of the image.
- height
- Type:object
- Optional
- The height of the image.
Code Sample
var pngImage = $(".selector").igMap("exportImage", 200, 100);
-
exportVisualData
- .igMap( "exportVisualData" );
Exports visual data from the map to aid in unit testing.
Code Sample
var data = $(".selector").igMap("exportVisualData");
-
flush
- .igMap( "flush" );
Forces any pending deferred work to render on the map before continuing.
Code Sample
$(".selector").igMap("flush");
-
getActualMaximumValue
- .igMap( "getActualMaximumValue", targetName:object );
Gets the actual maximum value of the target xAxis or yAxis.
- targetName
- Type:object
Code Sample
// Get maximum value for geographic latitude var maxLat = $(".selector").igMap("getActualMaximumValue", "yAxis"); // Get maximum value for geographic longitude var maxLon = $(".selector").igMap("getActualMaximumValue", "xAxis");
-
getActualMinimumValue
- .igMap( "getActualMinimumValue", targetName:object );
Gets the actual minimum value of the target xAxis or yAxis.
- targetName
- Type:object
Code Sample
// Get minimum value for geographic latitude var maxLat = $(".selector").igMap("getActualMinimumValue", "yAxis"); // Get minimum value for geographic longitude var maxLon = $(".selector").igMap("getActualMinimumValue", "xAxis");
-
getGeographicFromZoom
- .igMap( "getGeographicFromZoom", rect:object );
- Return Type:
- object
- Return Type Description:
- The window zoom area.
Given the current plot area of the control and a geographic region, get the WindowRect that would encompass that geographic region.
- rect
- Type:object
- The geographic area rectangle.
Code Sample
var geographic = $(".selector").igMap("getGeographicFromZoom", { left: 0, top: 0, width: 0.5, height: 0.5 });
-
getZoomFromGeographic
- .igMap( "getZoomFromGeographic", rect:object );
- Return Type:
- object
- Return Type Description:
- The window zoom area.
Given the current plot area of the control and a geographic region, get the WindowRect that would encompass that geographic region.
- rect
- Type:object
- The geographic area rectangle.
Code Sample
var zoom = $(".selector").igMap("getZoomFromGeographic", { left: 10, top: 10, width: 3, height: 3 });
-
id
- .igMap( "id" );
- Return Type:
- string
Returns the ID of parent element holding the map.
Code Sample
var dataContainerElement = $(".selector").igMap("id");
-
insertItem
- .igMap( "insertItem", item:object, index:number, targetName:string );
Inserts a new item to the data source and notifies the map.
- item
- Type:object
- the new item that we want to insert in the data source. .
- index
- Type:number
- The index in the data source where the new item will be inserted.
- targetName
- Type:string
- The name of the series bound to the data source.
Code Sample
$(".selector").igMap("insertItem", {"Latitude": 42.2, "Longitude": 22.5 }, 1, "series1" );
-
notifyClearItems
- .igMap( "notifyClearItems", dataSource:object );
- Return Type:
- object
- Return Type Description:
- Returns a reference to this igMap.
Notifies the the map that the items have been cleared from an associated data source.
It's not necessary to notify more than one target of a change if they share the same items source.- dataSource
- Type:object
- The data source in which the change happened.
Code Sample
var response = $(".selector").igMap("notifyClearItems", series1DataSource);
-
notifyContainerResized
- .igMap( "notifyContainerResized" );
Notifies the map that the container was resized.
Code Sample
$(".selector").igMap("notifyContainerResized");
-
notifyInsertItem
- .igMap( "notifyInsertItem", dataSource:object, index:number, newItem:object );
- Return Type:
- object
- Return Type Description:
- Returns a reference to this igMap.
Notifies the the target series that an item has been inserted at the specified index in its data source.
It's not necessary to notify more than one target of a change if they share the same items source.- dataSource
- Type:object
- The data source in which the change happened.
- index
- Type:number
- The index in the items source where the new item has been inserted.
- newItem
- Type:object
- The new item that has been set in the collection.
Code Sample
var response = $(".selector").igMap("notifyInsertItem", series1DataSource, 1, {"Latitude": 42.2, "Longitude": 22.5 });
-
notifyRemoveItem
- .igMap( "notifyRemoveItem", dataSource:object, index:number, oldItem:object );
- Return Type:
- object
- Return Type Description:
- Returns a reference to this igMap.
Notifies the the target series that an item has been removed from the specified index in its data source.
It's not necessary to notify more than one target of a change if they share the same items source.- dataSource
- Type:object
- The data source in which the change happened.
- index
- Type:number
- The index in the items source from where the old item has been removed.
- oldItem
- Type:object
- The old item that has been removed from the collection.
Code Sample
var response = $(".selector").igMap("notifyRemoveItem", series1DataSource, 1, {"Latitude": 42.2, "Longitude": 22.5 });
-
notifySetItem
- .igMap( "notifySetItem", dataSource:object, index:number, newItem:object, oldItem:object );
- Return Type:
- object
- Return Type Description:
- Returns a reference to this igMap.
Notifies the the map that an item has been set in an associated data source.
- dataSource
- Type:object
- The data source in which the change happened.
- index
- Type:number
- The index in the items source that has been changed.
- newItem
- Type:object
- The new item that has been set in the collection.
- oldItem
- Type:object
- The old item that has been overwritten in the collection.
Code Sample
var response = $(".selector").igMap("notifySetItem", series1DataSource, 1, {"Latitude": 42.2, "Longitude": 22.5 });
-
option
- .igMap( "option", key:object, value:object );
- key
- Type:object
- value
- Type:object
-
print
- .igMap( "print" );
Creates a print preview page with the map, hiding all other elements on the page.
Code Sample
$(".selector").igMap("print");
-
removeItem
- .igMap( "removeItem", index:number, targetName:string );
Deletes an item from the data source and notifies the map.
- index
- Type:number
- The index in the data source from where the item will be been removed.
- targetName
- Type:string
- The name of the series bound to the data source.
Code Sample
$(".selector").igMap("removeItem", 1, "series1");
-
renderSeries
- .igMap( "renderSeries", targetName:string, animate:bool );
Indicates that a series should render, even though no option has been modified that would normally cause it to refresh.
- targetName
- Type:string
- The name of the series to render.
- animate
- Type:bool
- Whether the change should be animated, if possible.
Code Sample
$(".selector").igMap("renderSeries", "xAxis", true);
-
resetZoom
- .igMap( "resetZoom" );
- Return Type:
- object
- Return Type Description:
- Returns a reference to this igMap.
Resets the zoom level of the map to default.
Code Sample
var response = $(".selector").igMap("resetZoom");
-
scaleValue
- .igMap( "scaleValue", targetName:string, unscaledValue:number );
- Return Type:
- number
- Return Type Description:
- Returns the scaled value.
Either xAxis or yAxis (longitude or latitude) that it should scale the requested value into map space from axis space.
For example you can use this method if you want to find where longitude 50 stands scaled to map's width.- targetName
- Type:string
- Either xAxis or yAxis to notify.
- unscaledValue
- Type:number
- The value in axis space to translate into map space.
Code Sample
var scaled = $(".selector").igMap("scaleValue", "series1", 250);
-
scrollIntoView
- .igMap( "scrollIntoView", targetName:string, item:object );
- Return Type:
- object
- Return Type Description:
- Returns a reference to this igMap.
Notifies the target series or axis that it should scroll the requested data item into view.
- targetName
- Type:string
- The name of the series or axis notify.
- item
- Type:object
- The data item to bring into view, if possible.
Code Sample
var response = $(".selector").igMap("scrollIntoView", 5);
-
setItem
- .igMap( "setItem", index:number, item:object, targetName:string );
Updates an item in the data source and notifies the map.
- index
- Type:number
- The index of the item in the data source that we want to change.
- item
- Type:object
- The new item object that will be set in the data source.
- targetName
- Type:string
- The name of the series bound to the data source.
Code Sample
$(".selector").igMap("setItem", 5, {"Latitude": 42.2, "Longitude": 22.5 }, "series1");
-
styleUpdated
- .igMap( "styleUpdated" );
- Return Type:
- object
- Return Type Description:
- Returns a reference to this igMap.
Notify the map that styles it draws colors from may have been updated.
Code Sample
var response = $(".selector").igMap("styleUpdated");
-
unscaleValue
- .igMap( "unscaleValue", targetName:string, scaledValue:number );
- Return Type:
- number
- Return Type Description:
- Returns the unscaled value.
Either xAxis or yAxis (longitude or latitude) that it should unscale the requested value into axis space from map space.
For example you can use this method if you want to find what is the longitude unscaled from 0 width of the map.- targetName
- Type:string
- Either xAxis or yAxis to notify.
- scaledValue
- Type:number
- The value in map space to translate into axis space.
Code Sample
var unscaled = $(".selector").igMap("unscaleValue", "series1", 50);
-
zoomToGeographic
- .igMap( "zoomToGeographic", rect:object );
- Return Type:
- object
- Return Type Description:
- Returns a reference to this igMap.
Zoom in to the geographic region specified, when possible (may need to wait fior map to be initialized).
- rect
- Type:object
- The geographic area rectangle.
-
ui-corner-all ui-widget-content
- Get or set the class applied on a div element.
-
ui-chart-tooltip ui-widget-content ui-corner-all
- Get or set the class applied to the tooltip div element.
-
ui-chart-non-html5-supported-message ui-helper-clearfix
- Get or set the class applied on a div element, shown when the map is opened in a non HTML5 compatible browser.