ui.igGridFiltering
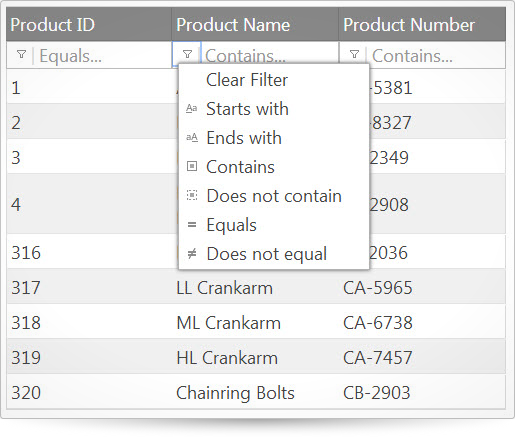
Both the igGrid and igHierarchicalGrid controls feature row filtering which allows users to restrict grid rows to only the data that meets a given criteria. Filtering options include numeric, string and date filters. Further information regarding the classes, options, events, methods and themes of this API are available under the associated tabs above.
The following code snippet demonstrates how to initialize the igGrid control.
Click here for more information on how to get started using this API. For details on how to reference the required scripts and themes for the igGrid control read, Using JavaScript Resources in Ignite UI and Styling and Theming Ignite UI.Code Sample
<!doctype html> <html> <head> <!-- Infragistics Combined CSS --> <link href="css/themes/infragistics/infragistics.theme.css" rel="stylesheet" type="text/css" /> <link href="css/structure/infragistics.css" rel="stylesheet" type="text/css" /> <!-- jQuery Core --> <script src="js/jquery.js" type="text/javascript"></script> <!-- jQuery UI --> <script src="js/jquery-ui.js" type="text/javascript"></script> <!-- Infragistics Combined Scripts --> <script src="js/infragistics.core.js" type="text/javascript"></script> <script src="js/infragistics.lob.js" type="text/javascript"></script> <script type="text/javascript"> var products = [ { "ProductID": 1, "Name": "Adjustable Race", "ProductNumber": "AR-5381" }, { "ProductID": 2, "Name": "Bearing Ball", "ProductNumber": "BA-8327" }, { "ProductID": 3, "Name": "BB Ball Bearing", "ProductNumber": "BE-2349" }, { "ProductID": 4, "Name": "Headset Ball Bearings", "ProductNumber": "BE-2908" }, { "ProductID": 316, "Name": "Blade", "ProductNumber": "BL-2036" }, { "ProductID": 317, "Name": "LL Crankarm", "ProductNumber": "CA-5965" }, { "ProductID": 318, "Name": "ML Crankarm", "ProductNumber": "CA-6738" }, { "ProductID": 319, "Name": "HL Crankarm", "ProductNumber": "CA-7457" }, { "ProductID": 320, "Name": "Chainring Bolts", "ProductNumber": "CB-2903" } ]; $(function () { $("#gridFiltering").igGrid({ columns: [ { headerText: "Product ID", key: "ProductID", dataType: "number" }, { headerText: "Product Name", key: "Name", dataType: "string" }, { headerText: "Product Number", key: "ProductNumber", dataType: "string" } ], features:[ { name: "Filtering" } ], width: "500px", dataSource: products }); }); </script> </head> <body> <table id="gridFiltering"></table> </body> </html>
Related Samples
Related Topics
Dependencies
Inherits
-
advancedModeEditorsVisible
- Type:
- bool
- Default:
- false
Defines whether to render editors in advanced mode. If false, no editors will be rendered in the advanced mode.
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", advancedModeEditorsVisible : true } ] }); // Get var editorsVisible = $(".selector").igGridFiltering("option", "advancedModeEditorsVisible"); // Set $(".selector").igGridFiltering("option", "advancedModeEditorsVisible", true);
-
advancedModeHeaderButtonLocation
- Type:
- enumeration
- Default:
- left
Location of the advanced filtering button when advancedModeEditorsVisible is false (i.e. when the button is rendered in the header).
Members
- left
- Type:string
- right
- Type:string
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", advancedModeHeaderButtonLocation : "right" } ] }); // Get var location = $(".selector").igGridFiltering("option", "advancedModeHeaderButtonLocation"); // Set $(".selector").igGridFiltering("option", "advancedModeHeaderButtonLocation", "right");
-
caseSensitive
- Type:
- bool
- Default:
- false
Enables or disables the filtering case sensitivity. Works only for local filtering. If true, it case sensitive filtering is performed. If false, filtering is case insensitive.
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", caseSensitive : true } ] }); // Get var caseSensitive = $(".selector").igGridFiltering("option", "caseSensitive"); // Set $(".selector").igGridFiltering("option", "caseSensitive", true);
-
columnSettings
- Type:
- array
- Default:
- []
- Elements Type:
- object
A list of column settings that specifies custom filtering options on a per column basis.
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", columnSettings : [ { columnKey: "ProductDescription", condition: "endsWith" } ] } ] }); // Get var colSettings = $(".selector").igGridFiltering("option", "columnSettings"); // Set $(".selector").igGridFiltering("option", "columnSettings", [{columnKey: "ProductDescription", condition: "endsWith" }] );
-
allowFiltering
- Type:
- bool
- Default:
- true
Enables/disables filtering for the column.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", columnSettings : [ { columnKey: "ProductDescription", allowFiltering: false } ] } ] }); //Get var colSettings = $(".selector").igGridFiltering("option", "columnSettings"); var filterAllowed = colSettings[0].allowFiltering; // Set $(".selector").igGridFiltering("option", "columnSettings", colSettings);
-
columnIndex
- Type:
- number
- Default:
- null
Identifies the grid column by index. Either key or index must be set in every column setting.
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", columnSettings : [ { columnIndex: 0, allowFiltering: false } ] } ] }); // Get var colSettings = $(".selector").igGridFiltering("option", "columnSettings"); var colIndex = colSettings[0].columnIndex;
-
columnKey
- Type:
- string
- Default:
- null
Identifies the grid column by key. Either key or index must be set in every column setting.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", columnSettings : [ { columnKey: "ProductDescription", allowFiltering: false } ] } ] }); //Get var colSettings = $(".selector").igGridFiltering("option", "columnSettings"); var colKey = colSettings[0].columnKey;
-
condition
- Type:
- enumeration
- Default:
- null
Initial filtering condition for the column.
Members
- empty
- Type:string
- notEmpty
- Type:string
- null
- Type:string
- notNull
- Type:string
- equals
- Type:string
- doesNotEqual
- Type:string
- startsWith
- Type:string
- contains
- Type:string
- doesNotContain
- Type:string
- endsWith
- Type:string
- greaterThan
- Type:string
- lessThan
- Type:string
- greaterThanOrEqualTo
- Type:string
- lessThanOrEqualTo
- Type:string
- true
- Type:bool
- false
- Type:bool
- on
- Type:string
- notOn
- Type:string
- before
- Type:string
- after
- Type:string
- today
- Type:string
- yesterday
- Type:string
- thisMonth
- Type:string
- lastMonth
- Type:string
- nextMonth
- Type:string
- thisYear
- Type:string
- nextYear
- Type:string
- lastYear
- Type:string
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", columnSettings : [ { columnKey: "ProductDescription", condition: "startsWith" } ] } ] }); // Get var colSettings = $(".selector").igGridFiltering("option", "columnSettings"); var condition = colSettings[0].condition; // Set $(".selector").igGridFiltering("option", "columnSettings", colSettings);
-
conditionList
- Type:
- array
- Default:
- []
- Elements Type:
- object
An array of strings that determine which conditions to display for this column.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", columnSettings : [{ columnKey: "ProductNumber", conditionList: ["startsWith", "contains"] }] } ] }); //Get var conditionList = $(".selector").igGridFiltering("option", "columnSettings")[2].conditionList;
-
customConditions
- Type:
- object
- Default:
- null
An object used to specify custom filtering conditions as objects for this column.
labelText The label as it will appear in the column's condition dropdown.
expressionText The text to display in the editor when requireExpr is false.
requireExpr If this condition requires the user to input a filtering expression.
filterImgIcon Class applied to the dropdown item when in simple mode.
filterFunc The custom comparing filter function. Signature: function (value, expression, dataType, ignoreCase, preciseDateFormat).Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", columnSettings : [{ columnKey: "ProductNumber", customConditions: { BE: { labelText: "BE", expressionText: "BE-####", requireExpr: false, filterFunc: filterProductNumber }, CA: { labelText: "CA", expressionText: "CA-####", requireExpr: false, filterFunc: filterProductNumber1 } } }] } ] }); function filterProductNumber(value, expression, dataType, ignoreCase, preciseDateFormat) { return value.startsWith("BE"); } function filterProductNumber1(value, expression, dataType, ignoreCase, preciseDateFormat) { return value.startsWith("CA"); } // Get var customConditions = $(".selector").igGridFiltering("option", "columnSettings")[2].customConditions;
-
defaultExpressions
- Type:
- object
- Default:
- []
Initial filtering expressions - if set they will be applied on initialization together with the preset condition.
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", columnSettings : [ { columnKey: "Name", defaultExpressions: [ { expr: "B", cond: "startsWith" } ] } ] } ] }); // Get var defaultExpressions = $(".selector").igGridFiltering("option", "columnSettings")[0].defaultExpressions;
-
dialogWidget
- Type:
- string
- Default:
- "igGridModalDialog"
Name of the dialog widget to be used. It should inherit from $.ui.igGridModalDialog.
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", dialogWidget: "advancedModalDialog" } ] }); // Get var widgetName = $(".selector").igGridFiltering("option", "dialogWidget");
-
featureChooserText
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Feature chooser text when filter is shown and filter mode is simple. Use option locale.featureChooserText.Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", featureChooserText : "Hide Filter" } ] }); // Get var text = $(".selector").igGridFiltering("option", "featureChooserText"); // Set $(".selector").igGridFiltering("option", "featureChooserText", "Hide Filter");
-
featureChooserTextAdvancedFilter
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Feature chooser text when filter mode is advanced. Use option locale.featureChooserTextAdvancedFilter.Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", featureChooserTextAdvancedFilter : "Advanced Filter" } ] }); // Get var text = $(".selector").igGridFiltering("option", "featureChooserTextAdvancedFilter"); // Set $(".selector").igGridFiltering("option", "featureChooserTextAdvancedFilter", "Advanced Filter");
-
featureChooserTextHide
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Feature chooser text when filter is hidden and filter mode is simple. Use option locale.featureChooserTextHide.Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", featureChooserTextHide : "Show Filter" } ] }); // Get var text = $(".selector").igGridFiltering("option", "featureChooserTextHide"); // Set $(".selector").igGridFiltering("option", "featureChooserTextHide", "Show Filter");
-
filterButtonLocation
- Type:
- enumeration
- Default:
- left
The filtering button for filter dropdowns can be rendered either on the left of the filter editor, or on the right.
Members
- left
- Type:string
- The button is rendered on the left.
- right
- Type:string
- The button is rendered on the right.
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterButtonLocation : "right" } ] }); // Get var location = $(".selector").igGridFiltering("option", "filterButtonLocation"); // Set $(".selector").igGridFiltering("option", "filterButtonLocation", "right");
-
filterDelay
- Type:
- number
- Default:
- 500
Time in milliseconds for which widget will wait between keystrokes before sending filtering requests.
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDelay : 1000 } ] }); // Get var delay = $(".selector").igGridFiltering("option", "filterDelay"); // Set $(".selector").igGridFiltering("option", "filterDelay", 1000);
-
filterDialogAddButtonWidth
- Type:
- enumeration
- Default:
- 100
Add button width - in the advanced filter dialog.
Members
- string
- Type:string
- The dialog Add button width in pixels (100px).
- number
- Type:number
- The dialog Add button width in pixels as a number (100).
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDialogAddButtonWidth : 75 } ] }); // Get var width = $(".selector").igGridFiltering("option", "filterDialogAddButtonWidth"); // Set $(".selector").igGridFiltering("option", "filterDialogAddButtonWidth", 75);
-
filterDialogAddConditionDropDownTemplate
- Type:
- string
- Default:
- null
Custom template for options in dropdown in add condition area in the filter dialog. The default template is "<option value='${value}'>${text}</option>".
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDialogAddConditionDropDownTemplate: "<option value='${text}'>${text}</option>" } ] }); // Get var dropDownTemplate = $(".selector").igGridFiltering("option", "filterDialogAddConditionDropDownTemplate"); // Set $(".selector").igGridFiltering("option", "filterDialogAddConditionDropDownTemplate", "<option value='${text}'>${text}</option>");
-
filterDialogAddConditionTemplate
- Type:
- string
- Default:
- null
Custom template for add condition area in the filter dialog. The default template is "<div><span>${label1}</span><div><select></select></div><span>${label2}</span></div>".
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDialogAddConditionTemplate: "<div><div><select></select></div><span>${label1}</span><span>${label2}</span></div>" } ] }); // Get var addConditionTemplate = $(".selector").igGridFiltering("option", "filterDialogAddConditionTemplate"); // Set $(".selector").igGridFiltering("option", "filterDialogAddConditionTemplate", "<div><div><select></select></div><span>${label1}</span><span>${label2}</span></div>");
-
filterDialogColumnDropDownDefaultWidth
- Type:
- enumeration
- Default:
- null
Width of the column chooser dropdowns in the advanced filter dialog.
Members
- string
- Type:string
- The column chooser dropdowns width in pixels (80px).
- number
- Type:number
- The column chooser dropdowns width in pixels as a number (80).
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDialogColumnDropDownDefaultWidth : 100 } ] }); // Get var width = $(".selector").igGridFiltering("option", "filterDialogColumnDropDownDefaultWidth"); // Set $(".selector").igGridFiltering("option", "filterDialogColumnDropDownDefaultWidth", 100);
-
filterDialogContainment
- Type:
- string
- Default:
- "owner"
Controls containment behavior.
owner The filter dialog will be draggable only within the grid area.
window The filter dialog will be draggable within the whole window area.Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDialogContainment : "window" } ] }); // Get var filterDialogContainment = $(".selector").igGridFiltering("option", "filterDialogContainment");
-
filterDialogExprInputDefaultWidth
- Type:
- enumeration
- Default:
- 130
Width of the filtering expression input boxes in the advanced filter dialog.
Members
- string
- Type:string
- The filtering expression input boxes width in pixels (80px).
- number
- Type:number
- The filtering expression input boxes width in pixels as a number (80).
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDialogExprInputDefaultWidth : 100 } ] }); // Get var width = $(".selector").igGridFiltering("option", "filterDialogExprInputDefaultWidth"); // Set $(".selector").igGridFiltering("option", "filterDialogExprInputDefaultWidth", 100);
-
filterDialogFilterConditionTemplate
- Type:
- string
- Default:
- null
Custom template for options in condition list in filter dialog. The default template is "<option value='${condition}'>${text}</option>".
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDialogFilterConditionTemplate: "<option value='${conditionName}'>${conditionLabel}</option>" } ] }); // Get var filterConditionTemplate = $(".selector").igGridFiltering("option", "filterDialogFilterConditionTemplate"); // Set $(".selector").igGridFiltering("option", "filterDialogFilterConditionTemplate", "<option value='${conditionName}'>${conditionLabel}</option>");
-
filterDialogFilterDropDownDefaultWidth
- Type:
- enumeration
- Default:
- 80
Width of the filtering condition dropdowns in the advanced filter dialog.
Members
- string
- Type:string
- The filtering condition dropdowns width in pixels (80px).
- number
- Type:number
- The filtering condition dropdowns width in pixels as a number (80).
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDialogFilterDropDownDefaultWidth : 100 } ] }); // Get var width = $(".selector").igGridFiltering("option", "filterDialogFilterDropDownDefaultWidth"); // Set $(".selector").igGridFiltering("option", "filterDialogFilterDropDownDefaultWidth", 100);
-
filterDialogFilterTemplate
- Type:
- string
- Default:
- null
Custom template for filter dialog.
Each DOM element which is used for selecting filter conditions/columns/filter expressions has "data-*" attribute.
E.g.: DOM element used for selecting column has attribute "data-af-col", for selecting filter condition - "data-af-cond", for filter expression- "data-af-expr".
NOTE: The template is supported only with <tr />.
The default template is "<tr data-af-row><td><input data-af-col/></td><td><select data-af-cond></select></td><td><input data-af-expr /> </td><td><span data-af-rmv></span></td></tr>".Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDialogFilterTemplate: "<tr><td>Choose column<input/></td><td>Condition<select></select></td><td>Search value<input /> </td><td><span></span></td></tr>" } ] }); // Get var dialogFilterTemplate = $(".selector").igGridFiltering("option", "filterDialogFilterTemplate"); // Set $(".selector").igGridFiltering("option", "filterDialogFilterTemplate", "<tr><td>Choose column<input/></td><td>Condition<select></select></td><td>Search value<input /> </td><td><span></span></td></tr>");
-
filterDialogHeight
- Type:
- enumeration
- Default:
- ""
default filter dialog height (used for Advanced filtering mode).
Members
- string
- Type:string
- The dialog window height in pixels (350px).
- number
- Type:number
- The dialog window height in pixels as a number (350).
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDialogHeight : 30 } ] }); // Get var height = $(".selector").igGridFiltering("option", "filterDialogHeight"); // Set $(".selector").igGridFiltering("option", "filterDialogHeight", 30);
-
filterDialogMaxFilterCount
- Type:
- number
- Default:
- 5
Maximum number of filter rows in the advanced filtering dialog. If this number is exceeded, an error message will be rendered.
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDialogMaxFilterCount : 3 } ] }); // Get var count = $(".selector").igGridFiltering("option", "filterDialogMaxFilterCount"); // Set $(".selector").igGridFiltering("option", "filterDialogMaxFilterCount", 3);
-
filterDialogOkCancelButtonWidth
- Type:
- enumeration
- Default:
- 120
Width of the Ok and Cancel buttons in the advanced filtering dialogs.
Members
- string
- Type:string
- The advanced filter dialog Ok and Cancel buttons width in pixels (120px).
- number
- Type:number
- The advanced filter dialog Ok and Cancel buttons width in pixels as a number (120).
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDialogOkCancelButtonWidth : 120 } ] }); // Get var width = $(".selector").igGridFiltering("option", "filterDialogOkCancelButtonWidth"); // Set $(".selector").igGridFiltering("option", "filterDialogOkCancelButtonWidth", 120);
-
filterDialogWidth
- Type:
- enumeration
- Default:
- 430
Default filter dialog width (used for Advanced filtering mode).
Members
- string
- Type:string
- The dialog window width in pixels (370px).
- number
- Type:number
- The dialog window width in pixels as a number (370).
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDialogWidth : 500 } ] }); // Get var width = $(".selector").igGridFiltering("option", "filterDialogWidth"); // Set $(".selector").igGridFiltering("option", "filterDialogWidth", 500);
-
filterDropDownAnimationDuration
- Type:
- number
- Default:
- 500
Animation duration in milliseconds for the filterDropDownAnimations.
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDropDownAnimationDuration : 300 } ] }); // Get var duration = $(".selector").igGridFiltering("option", "filterDropDownAnimationDuration"); // Set $(".selector").igGridFiltering("option", "filterDropDownAnimationDuration", 300);
-
filterDropDownAnimations
- Type:
- enumeration
- Default:
- linear
Type of animations for the column filter dropdowns.
Members
- linear
- Type:string
- The column filtering drop downs are shown with a linear animation.
- none
- Type:string
- No animation is used when showing the filtering drop downs.
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDropDownAnimations : "none" } ] }); // Get var animation = $(".selector").igGridFiltering("option", "filterDropDownAnimations"); // Set $(".selector").igGridFiltering("option", "filterDropDownAnimations", "none");
-
filterDropDownHeight
- Type:
- object
- Default:
- 0
Height of the column filter dropdowns.
string The height of the column filter dropdowns in pixels (0px).
number The height of the column filter dropdowns in pixels as a number (0).Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDropDownHeight : 30 } ] }); // Get var height = $(".selector").igGridFiltering("option", "filterDropDownHeight"); // Set $(".selector").igGridFiltering("option", "filterDropDownHeight", 30);
-
filterDropDownItemIcons
- Type:
- enumeration
- Default:
- true
Enable/disable filter icons visibility.
Members
- true
- Type:bool
- All predefined filters in the filter dropdowns will have icons rendered in front of the text.
- false
- Type:bool
- No icons will be rendered.
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDropDownItemIcons : false } ] }); // Get var showIcons = $(".selector").igGridFiltering("option", "filterDropDownItemIcons"); // Set $(".selector").igGridFiltering("option", "filterDropDownItemIcons", false);
-
filterDropDownWidth
- Type:
- enumeration
- Default:
- 0
Width of the column filter dropdowns.
Members
- string
- Type:string
- The width in pixels (0px).
- number
- Type:number
- The width in pixels as a number (0).
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDropDownWidth : 100 } ] }); // Get var width = $(".selector").igGridFiltering("option", "filterDropDownWidth"); // Set $(".selector").igGridFiltering("option", "filterDropDownWidth", 100);
-
filterExprUrlKey
- Type:
- string
- Default:
- null
URL key name that specifies how the filtering expressions will be encoded for remote requests, e.g. &filter('col') = startsWith. Default is OData.
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterExprUrlKey : "filter" } ] }); // Get var key = $(".selector").igGridFiltering("option", "filterExprUrlKey"); // Set $(".selector").igGridFiltering("option", "filterExprUrlKey", "filter");
-
filterSummaryAlwaysVisible
- Type:
- bool
- Default:
- true
Enable/disable footer visibility with summary info about the filter.
When false, the filter summary row (in the footer) will only be visible when paging is enabled (or some other feature that renders a footer).
When true, the filter summary row will only be visible when a filter is applied i.e. it's not visible by default.Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterSummaryAlwaysVisible : false } ] }); // Get var showSummary = $(".selector").igGridFiltering("option", "filterSummaryAlwaysVisible"); // Set $(".selector").igGridFiltering("option", "filterSummaryAlwaysVisible", false);
-
filterSummaryTemplate
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Summary template that will appear in the bottom left corner of the footer. Has the format '${matches} matching records'. Use option locale.filterSummaryTemplate.Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterSummaryTemplate: "${matches} products found" } ] }); // Get var summaryTemplate = $(".selector").igGridFiltering("option", "filterSummaryTemplate"); // Set $(".selector").igGridFiltering("option", "filterSummaryTemplate", "${matches} products");
-
inherit
- Type:
- bool
- Default:
- false
Enables/disables feature inheritance for the child layouts. NOTE: It only applies for igHierarchicalGrid.
Code Sample
// Initialize $(".selector").igHierarchicalGrid({ features: [ { name: "Filtering", inherit: true } ] }); // Get var inherit = $(".selector").igGridFiltering("option", "inherit");
-
labels
Removed- Type:
- string
- Default:
- "{}"
This option has been removed as of 2017.2 Volume release.
A list of configurable and localized labels that are used for the predefined filtering conditions in the filter dropdowns. Use option locale.Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { advancedButtonLabel: "Advanced", after: "After", before: "Before", clear: "Clear Filter", contains: "Contains" //... } } ] }); // Get var filteringLabels = $(".selector").igGridFiltering("option", "labels");
-
advancedButtonLabel
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { advancedButtonLabel: "Advanced" } } ] });
-
after
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { after: "after" } } ] });
-
before
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { before: "before" } } ] });
-
clear
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { clear: "clear filter" } } ] });
-
contains
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { contains: "contains" } } ] });
-
doesNotContain
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { doesNotContain: "does Not contain" } } ] });
-
doesNotEqual
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { doesNotEqual: "does Not equal" } } ] });
-
empty
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { empty: "empty" } } ] });
-
endsWith
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { endsWith: "ends with" } } ] });
-
equals
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { equals: "equals" } } ] });
-
falseLabel
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { falseLabel: "false" } } ] });
-
filterDialogAddLabel
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { filterDialogAddLabel: "add" } } ] });
-
filterDialogAllLabel
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { filterDialogAllLabel: "all" } } ] });
-
filterDialogAnyLabel
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { filterDialogAnyLabel: "any" } } ] });
-
filterDialogCancelLabel
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { filterDialogCancelLabel: "cancel" } } ] });
-
filterDialogCaptionLabel
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { filterDialogCaptionLabel: "Advanced Filtering" } } ] });
-
filterDialogClearAllLabel
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { filterDialogClearAllLabel: "clear all" } } ] });
-
filterDialogConditionLabel1
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { filterDialogConditionLabel1: "Show" } } ] });
-
filterDialogConditionLabel2
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { filterDialogConditionLabel2: "records matching the following criteria" } } ] });
-
filterDialogErrorLabel
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { filterDialogErrorLabel: "error" } } ] });
-
filterDialogOkLabel
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { filterDialogOkLabel: "ok" } } ] });
-
filterSummaryTitleLabel
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { filterSummaryTitleLabel: "filtering summary" } } ] });
-
greaterThan
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { greaterThan: "greater than" } } ] });
-
greaterThanOrEqualTo
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { greaterThanOrEqualTo: "greater than or equal to" } } ] });
-
lastMonth
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { lastMonth: "last month" } } ] });
-
lastYear
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { lastYear: "last year" } } ] });
-
lessThan
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { lessThan: "less than" } } ] });
-
lessThanOrEqualTo
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { lessThanOrEqualTo: "less than or equal to" } } ] });
-
nextMonth
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { nextMonth: "next month" } } ] });
-
nextYear
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { nextYear: "next year" } } ] });
-
noFilter
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { noFilter: "no filter" } } ] });
-
notEmpty
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { notEmpty: "not empty" } } ] });
-
notNull
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { notNull: "not null" } } ] });
-
notOn
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { notOn: "not on" } } ] });
-
nullLabel
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { nullLabel: "null label" } } ] });
-
on
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { on: "on" } } ] });
-
startsWith
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { startsWith: "starts with" } } ] });
-
thisMonth
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { thisMonth: "this month" } } ] });
-
thisYear
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { thisYear: "this year" } } ] });
-
today
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { today: "today" } } ] });
-
true
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { true: "True" } } ] });
-
trueLabel
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { trueLabel: "true" } } ] });
-
yesterday
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", labels: { yesterday: "yesterday" } } ] });
-
language
Inherited- Type:
- string
- Default:
- "en"
Set/Get the locale language setting for the widget.
Code Sample
//Initialize $(".selector").igGridFiltering({ language: "ja" }); // Get var language = $(".selector").igGridFiltering("option", "language"); // Set $(".selector").igGridFiltering("option", "language", "ja");
-
locale
- Type:
- object
- Default:
- {}
-
advancedButtonLabel
- Type:
- string
- Default:
- ""
'Advance Button' label that is used for the predefined filtering conditions in the filter dropdowns.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { advancedButtonLabel : "Advance Button"} } ] }); //Get var advancedButtonLabel = $(".selector").igGridFiltering("option", "locale").advancedButtonLabel; //Set $(".selector").igGridFiltering("option", "locale", {advancedButtonLabel : "Advance Button"});
-
afterLabel
- Type:
- string
- Default:
- ""
'After' label that is used for the predefined filtering conditions in the filter dropdowns.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { afterLabel : "After"} } ] }); //Get var afterLabel = $(".selector").igGridFiltering("option", "locale").afterLabel; //Set $(".selector").igGridFiltering("option", "locale", {afterLabel : "After"});
-
afterNullText
- Type:
- string
- Default:
- ""
After null text that will be used for the filter editors.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { afterNullText : "After"} } ] }); //Get var afterNullText = $(".selector").igGridFiltering("option", "locale").afterNullText; //Set $(".selector").igGridFiltering("option", "locale", {afterNullText : "After"});
-
beforeLabel
- Type:
- string
- Default:
- ""
'Before' label that is used for the predefined filtering conditions in the filter dropdowns.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { beforeLabel : "Before"} } ] }); //Get var beforeLabel = $(".selector").igGridFiltering("option", "locale").beforeLabel; //Set $(".selector").igGridFiltering("option", "locale", {beforeLabel : "Before"});
-
beforeNullText
- Type:
- string
- Default:
- ""
Before null text that will be used for the filter editors.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { beforeNullText : "Before"} } ] }); //Get var beforeNullText = $(".selector").igGridFiltering("option", "locale").beforeNullText; //Set $(".selector").igGridFiltering("option", "locale", {beforeNullText : "Before"});
-
clearLabel
- Type:
- string
- Default:
- ""
'Clear' label that is used for the predefined filtering conditions in the filter dropdowns.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { clearLabel : "Clear"} } ] }); //Get var clearLabel = $(".selector").igGridFiltering("option", "locale").clearLabel; //Set $(".selector").igGridFiltering("option", "locale", {clearLabel : "Clear"});
-
containsLabel
- Type:
- string
- Default:
- ""
'Contains' label that is used for the predefined filtering conditions in the filter dropdowns.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { containsLabel : "Contains"} } ] }); //Get var containsLabel = $(".selector").igGridFiltering("option", "locale").containsLabel; //Set $(".selector").igGridFiltering("option", "locale", {containsLabel : "Contains"});
-
containsNullText
- Type:
- string
- Default:
- ""
Contains null text that will be used for the filter editors.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { containsNullText : "Contains"} } ] }); //Get var endsWithNullText = $(".selector").igGridFiltering("option", "locale").containsNullText; //Set $(".selector").igGridFiltering("option", "locale", {containsNullText : "Contains"});
-
doesNotContainLabel
- Type:
- string
- Default:
- ""
'Does not contain' label that is used for the predefined filtering conditions in the filter dropdowns.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { doesNotContainLabel : "Does not contain"} } ] }); //Get var doesNotContainLabel = $(".selector").igGridFiltering("option", "locale").doesNotContainLabel; //Set $(".selector").igGridFiltering("option", "locale", {doesNotContainLabel : "Does not contain"});
-
doesNotContainNullText
- Type:
- string
- Default:
- ""
Does not contain null text that will be used for the filter editors.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { doesNotContainNullText : "Doesn't contain"} } ] }); //Get var doesNotContainNullText = $(".selector").igGridFiltering("option", "locale").doesNotContainNullText; //Set $(".selector").igGridFiltering("option", "locale", {doesNotContainNullText : "Doesn't contain"});
-
doesNotEqualLabel
- Type:
- string
- Default:
- ""
'Does not Equal' label that is used for the predefined filtering conditions in the filter dropdowns.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { doesNotEqualLabel : "Does not Equal"} } ] }); //Get var doesNotEqualLabel = $(".selector").igGridFiltering("option", "locale").doesNotEqualLabel; //Set $(".selector").igGridFiltering("option", "locale", {doesNotEqualLabel : "Does not Equal"});
-
doesNotEqualNullText
- Type:
- string
- Default:
- ""
Does not equal null text that will be used for the filter editors.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { doesNotEqualNullText : "Equals"} } ] }); //Get var doesNotEqualNullText = $(".selector").igGridFiltering("option", "locale").doesNotEqualNullText; //Set $(".selector").igGridFiltering("option", "locale", {doesNotEqualNullText : "Equals"});
-
emptyNullText
- Type:
- string
- Default:
- ""
Empty null text that will be used for the filter editors.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { beforeNullText : "Before"} } ] }); //Get var beforeNullText = $(".selector").igGridFiltering("option", "locale").beforeNullText; //Set $(".selector").igGridFiltering("option", "locale", {beforeNullText : "Before"});
-
endsWithLabel
- Type:
- string
- Default:
- ""
'Starts with' label that is used for the predefined filtering conditions in the filter dropdowns.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { endsWithLabel : "Starts With"} } ] }); //Get var endsWithLabel = $(".selector").igGridFiltering("option", "locale").endsWithLabel; //Set $(".selector").igGridFiltering("option", "locale", {endsWithLabel : "Ends With"});
-
endsWithNullText
- Type:
- string
- Default:
- ""
EndsWith null text that will be used for the filter editors.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { endsWithNullText : "Ends With"} } ] }); //Get var endsWithNullText = $(".selector").igGridFiltering("option", "locale").endsWithNullText; //Set $(".selector").igGridFiltering("option", "locale", {endsWithNullText : "Ends With"});
-
equalsLabel
- Type:
- string
- Default:
- ""
'Equals' label that is used for the predefined filtering conditions in the filter dropdowns.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { equalsLabel : "Equals"} } ] }); //Get var equalsLabel = $(".selector").igGridFiltering("option", "locale").equalsLabel; //Set $(".selector").igGridFiltering("option", "locale", {equalsLabel : "Equals"});
-
equalsNullText
- Type:
- string
- Default:
- ""
Equals null text that will be used for the filter editors.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { equalsNullText : "Equals"} } ] }); //Get var equalsNullText = $(".selector").igGridFiltering("option", "locale").equalsNullText; //Set $(".selector").igGridFiltering("option", "locale", {equalsNullText : "Equals"});
-
falseLabel
- Type:
- string
- Default:
- ""
'False' label that is used for the predefined filtering conditions in the filter dropdowns.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { falseLabel : "False"} } ] }); //Get var falseLabel = $(".selector").igGridFiltering("option", "locale").falseLabel; //Set $(".selector").igGridFiltering("option", "locale", {falseLabel : "False"});
-
featureChooserText
- Type:
- string
- Default:
- ""
Feature chooser text when filter is shown and filter mode is simple.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { featureChooserText : "Hide Filter"} } ] }); //Get var featureChooserText = $(".selector").igGridFiltering("option", "locale").featureChooserText; //Set $(".selector").igGridFiltering("option", "locale", {featureChooserText : "Hide Filter"});
-
featureChooserTextAdvancedFilter
- Type:
- string
- Default:
- ""
Feature chooser text when filter mode is advanced.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { featureChooserTextAdvancedFilter : "Advanced Filter"} } ] }); //Get var featureChooserTextAdvancedFilter = $(".selector").igGridFiltering("option", "locale").featureChooserTextAdvancedFilter; //Set $(".selector").igGridFiltering("option", "locale", {featureChooserTextAdvancedFilter : "Advanced Filter"});
-
featureChooserTextHide
- Type:
- string
- Default:
- ""
Feature chooser text when filter is hidden and filter mode is simple.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { featureChooserTextHide : "Hide Filter"} } ] }); //Get var featureChooserTextHide = $(".selector").igGridFiltering("option", "locale").featureChooserTextHide; //Set $(".selector").igGridFiltering("option", "locale", {featureChooserTextHide : "Hide Filter"});
-
filterDialogAddLabel
- Type:
- string
- Default:
- ""
Specifies the Add button label for the filtering dialog.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { filterDialogAddLabel : "Add"} } ] }); //Get var filterDialogAddLabel = $(".selector").igGridFiltering("option", "locale").filterDialogAddLabel; //Set $(".selector").igGridFiltering("option", "locale", {filterDialogAddLabel : "Add"});
-
filterDialogAllLabel
- Type:
- string
- Default:
- ""
Specifies the All label for the filtering dialog.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { filterDialogAllLabel : "All"} } ] }); //Get var filterDialogAllLabel = $(".selector").igGridFiltering("option", "locale").filterDialogAllLabel; //Set $(".selector").igGridFiltering("option", "locale", {filterDialogAllLabel : "All"});
-
filterDialogAnyLabel
- Type:
- string
- Default:
- ""
Specifies the Any label for the filtering dialog.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { filterDialogAnyLabel : "Any"} } ] }); //Get var filterDialogAnyLabel = $(".selector").igGridFiltering("option", "locale").filterDialogAnyLabel; //Set $(".selector").igGridFiltering("option", "locale", {filterDialogAnyLabel : "Any"});
-
filterDialogCancelLabel
- Type:
- string
- Default:
- ""
Specifies the dialog's Cancel button label.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { filterDialogCancelLabel : "Search"} } ] }); //Get var filterDialogCancelLabel = $(".selector").igGridFiltering("option", "locale").filterDialogCancelLabel; //Set $(".selector").igGridFiltering("option", "locale", {filterDialogCancelLabel : "Search"});
-
filterDialogCaptionLabel
- Type:
- string
- Default:
- ""
Specifies the filter dialog caption label.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { filterDialogCaptionLabel : "Label"} } ] }); //Get var filterDialogCaptionLabel = $(".selector").igGridFiltering("option", "locale").filterDialogCaptionLabel; //Set $(".selector").igGridFiltering("option", "locale", {filterDialogCaptionLabel : "Label"});
-
filterDialogClearAllLabel
- Type:
- string
- Default:
- ""
Specifies clear all label in the filter dialog.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { filterDialogClearAllLabel : "Clear ALL"} } ] }); //Get var filterDialogClearAllLabel = $(".selector").igGridFiltering("option", "locale").filterDialogClearAllLabel; //Set $(".selector").igGridFiltering("option", "locale", {filterDialogClearAllLabel : "Clear ALL"});
-
filterDialogCloseLabel
- Type:
- string
- Default:
- ""
Specifies the Close label for the filtering dialog.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { filterDialogCloseLabel : "Close"} } ] }); //Get var filterDialogCloseLabel = $(".selector").igGridFiltering("option", "locale").filterDialogCloseLabel; //Set $(".selector").igGridFiltering("option", "locale", {filterDialogCloseLabel : "Close"});
-
filterDialogConditionDropDownLabel
- Type:
- string
- Default:
- ""
Specifies the filter condition drop-down label.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { filterDialogConditionDropDownLabel : "Filtering condition"} } ] }); //Get var filterDialogConditionDropDownLabel = $(".selector").igGridFiltering("option", "locale").filterDialogConditionDropDownLabel; //Set $(".selector").igGridFiltering("option", "locale", {filterDialogConditionDropDownLabel : "Filtering condition"});
-
filterDialogConditionLabel1
- Type:
- string
- Default:
- ""
Specifies the filter condition label.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { filterDialogConditionLabel1 : "Show records matching"} } ] }); //Get var filterDialogConditionLabel1 = $(".selector").igGridFiltering("option", "locale").filterDialogConditionLabel1; //Set $(".selector").igGridFiltering("option", "locale", {filterDialogConditionLabel1 : "Show records matching"});
-
filterDialogConditionLabel2
- Type:
- string
- Default:
- ""
Specifies the filter condition label.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { filterDialogConditionLabel2 : " of the following criteria"} } ] }); //Get var filterDialogConditionLabel2 = $(".selector").igGridFiltering("option", "locale").filterDialogConditionLabel2; //Set $(".selector").igGridFiltering("option", "locale", {filterDialogConditionLabel2 : " of the following criteria"});
-
filterDialogErrorLabel
- Type:
- string
- Default:
- ""
Specifies the Error label for the filtering dialog.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { filterDialogErrorLabel : "You reached the maximum number of filters supported."} } ] }); //Get var filterDialogErrorLabel = $(".selector").igGridFiltering("option", "locale").filterDialogErrorLabel; //Set $(".selector").igGridFiltering("option", "locale", {filterDialogErrorLabel : "You reached the maximum number of filters supported."});
-
filterDialogOkLabel
- Type:
- string
- Default:
- ""
Specifies the dialog's Ok button label.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { filterDialogOkLabel : "Search"} } ] }); //Get var filterDialogOkLabel = $(".selector").igGridFiltering("option", "locale").filterDialogOkLabel; //Set $(".selector").igGridFiltering("option", "locale", {filterDialogOkLabel : "Search"});
-
filterSummaryTemplate
- Type:
- string
- Default:
- ""
Specifies the summary template for the matching records.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { filterSummaryTemplate : "${matches} matching records"} } ] }); //Get var filterSummaryTemplate = $(".selector").igGridFiltering("option", "locale").filterSummaryTemplate; //Set $(".selector").igGridFiltering("option", "locale", {filterSummaryTemplate : "${matches} matching records"});
-
filterSummaryTitleLabel
- Type:
- string
- Default:
- ""
Specifies the Filtering summary title.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { filterSummaryTitleLabel : "Search results"} } ] }); //Get var filterSummaryTitleLabel = $(".selector").igGridFiltering("option", "locale").filterSummaryTitleLabel; //Set $(".selector").igGridFiltering("option", "locale", {filterSummaryTitleLabel : "Search results"});
-
greaterThanLabel
- Type:
- string
- Default:
- ""
'Greater Than' label that is used for the predefined filtering conditions in the filter dropdowns.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { greaterThanLabel : "Greater Than"} } ] }); //Get var greaterThanLabel = $(".selector").igGridFiltering("option", "locale").greaterThanLabel; //Set $(".selector").igGridFiltering("option", "locale", {greaterThanLabel : "Greater Than"});
-
greaterThanNullText
- Type:
- string
- Default:
- ""
Greater than null text that will be used for the filter editors.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { greaterThanNullText : "Greater than"} } ] }); //Get var greaterThanNullText = $(".selector").igGridFiltering("option", "locale").greaterThanNullText; //Set $(".selector").igGridFiltering("option", "locale", {greaterThanNullText : "Greater than"});
-
greaterThanOrEqualToLabel
- Type:
- string
- Default:
- ""
'Greater Than or Equal' label that is used for the predefined filtering conditions in the filter dropdowns.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { greaterThanOrEqualToLabel : "Greater Than or Equal"} } ] }); //Get var greaterThanOrEqualToLabel = $(".selector").igGridFiltering("option", "locale").greaterThanOrEqualToLabel; //Set $(".selector").igGridFiltering("option", "locale", {greaterThanOrEqualToLabel : "Greater Than or Equal"});
-
greaterThanOrEqualToNullText
- Type:
- string
- Default:
- ""
Greater than or equal to null text that will be used for the filter editors.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { greaterThanOrEqualToNullText : "Greater Than or Equals to"} } ] }); //Get var greaterThanOrEqualToNullText = $(".selector").igGridFiltering("option", "locale").greaterThanOrEqualToNullText; //Set $(".selector").igGridFiltering("option", "locale", {greaterThanOrEqualToNullText : "Greater Than or Equals to"});
-
lastMonthLabel
- Type:
- string
- Default:
- ""
'Last Month' label that is used for the predefined filtering conditions in the filter dropdowns.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { lastMonthLabel : "Last Month"} } ] }); //Get var lastMonthLabel = $(".selector").igGridFiltering("option", "locale").lastMonthLabel; //Set $(".selector").igGridFiltering("option", "locale", {lastMonthLabel : "Last Month"});
-
lastYearLabel
- Type:
- string
- Default:
- ""
'Last Year' label that is used for the predefined filtering conditions in the filter dropdowns.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { lastYearLabel : "Last Year"} } ] }); //Get var lastYearLabel = $(".selector").igGridFiltering("option", "locale").lastYearLabel; //Set $(".selector").igGridFiltering("option", "locale", {lastYearLabel : "Last Year"});
-
lessThanLabel
- Type:
- string
- Default:
- ""
'Less Than' label that is used for the predefined filtering conditions in the filter dropdowns.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { lessThanLabel : "Less Than"} } ] }); //Get var lessThanLabel = $(".selector").igGridFiltering("option", "locale").lessThanLabel; //Set $(".selector").igGridFiltering("option", "locale", {lessThanLabel : "Less Than"});
-
lessThanNullText
- Type:
- string
- Default:
- ""
Less than null text that will be used for the filter editors.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { lessThanNullText : "Less Than Null"} } ] }); //Get var lessThanNullText = $(".selector").igGridFiltering("option", "locale").lessThanNullText; //Set $(".selector").igGridFiltering("option", "locale", {lessThanNullText : "Less Than Null"});
-
lessThanOrEqualToLabel
- Type:
- string
- Default:
- ""
'Less Than or Equal' label that is used for the predefined filtering conditions in the filter dropdowns.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { lessThanOrEqualToLabel : "Less Than or Equal"} } ] }); //Get var lessThanOrEqualToLabel = $(".selector").igGridFiltering("option", "locale").lessThanOrEqualToLabel; //Set $(".selector").igGridFiltering("option", "locale", {lessThanOrEqualToLabel : "Less Than or Equal"});
-
lessThanOrEqualToNullText
- Type:
- string
- Default:
- ""
Less than or equal to null text that will be used for the filter editors.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { lessThanOrEqualToNullText : "Less Than or Equals to"} } ] }); //Get var lessThanOrEqualToNullText = $(".selector").igGridFiltering("option", "locale").lessThanOrEqualToNullText; //Set $(".selector").igGridFiltering("option", "locale", {lessThanOrEqualToNullText : "Less Than or Equals to"});
-
nextMonthLabel
- Type:
- string
- Default:
- ""
'Next Month' label that is used for the predefined filtering conditions in the filter dropdowns.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { nextMonthLabel : "Next Month"} } ] }); //Get var nextMonthLabel = $(".selector").igGridFiltering("option", "locale").nextMonthLabel; //Set $(".selector").igGridFiltering("option", "locale", {nextMonthLabel : "Next Month"});
-
nextYearLabel
- Type:
- string
- Default:
- ""
'Next Year' label that is used for the predefined filtering conditions in the filter dropdowns.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { nextYearLabel : "Next Year"} } ] }); //Get var nextYearLabel = $(".selector").igGridFiltering("option", "locale").nextYearLabel; //Set $(".selector").igGridFiltering("option", "locale", {nextYearLabel : "Next Year"});
-
noFilterLabel
- Type:
- string
- Default:
- ""
'No Filter' label that is used for the predefined filtering conditions in the filter dropdowns.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { noFilterLabel : "No Filter"} } ] }); //Get var noFilterLabel = $(".selector").igGridFiltering("option", "locale").noFilterLabel; //Set $(".selector").igGridFiltering("option", "locale", {noFilterLabel : "No Filter"});
-
notEmptyNullText
- Type:
- string
- Default:
- ""
Not empty null text that will be used for the filter editors.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { notEmptyNullText : "Empty"} } ] }); //Get var notEmptyNullText = $(".selector").igGridFiltering("option", "locale").notEmptyNullText; //Set $(".selector").igGridFiltering("option", "locale", {notEmptyNullText : "Empty"});
-
notNullNullText
- Type:
- string
- Default:
- ""
Not empty null text that will be used for the filter editors.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { notNullNullText : "Not Null"} } ] }); //Get var notNullNullText = $(".selector").igGridFiltering("option", "locale").notNullNullText; //Set $(".selector").igGridFiltering("option", "locale", {notNullNullText : "Not Null"});
-
notOnLabel
- Type:
- string
- Default:
- ""
'Not On' label that is used for the predefined filtering conditions in the filter dropdowns.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { notOnLabel : "Not On"} } ] }); //Get var notOnLabel = $(".selector").igGridFiltering("option", "locale").notOnLabel; //Set $(".selector").igGridFiltering("option", "locale", {notOnLabel : "Not On"});
-
notOnNullText
- Type:
- string
- Default:
- ""
Not on null text that will be used for the filter editors.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { notOnNullText : "Not On"} } ] }); //Get var notOnNullText = $(".selector").igGridFiltering("option", "locale").notOnNullText; //Set $(".selector").igGridFiltering("option", "locale", {notOnNullText : "Not On"});
-
nullNullText
- Type:
- string
- Default:
- ""
Not empty null text that will be used for the filter editors.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { nullNullText : "Null"} } ] }); //Get var nullNullText = $(".selector").igGridFiltering("option", "locale").nullNullText; //Set $(".selector").igGridFiltering("option", "locale", {nullNullText : "Null"});
-
onLabel
- Type:
- string
- Default:
- ""
'On' label that is used for the predefined filtering conditions in the filter dropdowns.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { onLabel : "On"} } ] }); //Get var onLabel = $(".selector").igGridFiltering("option", "locale").onLabel; //Set $(".selector").igGridFiltering("option", "locale", {onLabel : "On"});
-
onNullText
- Type:
- string
- Default:
- ""
On null text that will be used for the filter editors.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { onNullText : "On"} } ] }); //Get var onNullText = $(".selector").igGridFiltering("option", "locale").onNullText; //Set $(".selector").igGridFiltering("option", "locale", {onNullText : "On"});
-
startsWithLabel
- Type:
- string
- Default:
- ""
'Starts with' label that is used for the predefined filtering conditions in the filter dropdowns.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { startsWithLabel : "Starts With"} } ] }); //Get var startsWithLabel = $(".selector").igGridFiltering("option", "locale").startsWithLabel; //Set $(".selector").igGridFiltering("option", "locale", {startsWithLabel : "Starts With"});
-
startsWithNullText
- Type:
- string
- Default:
- ""
StartsWith null text that will be used for the filter editors.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { startsWithNullText : "Starts With"} } ] }); //Get var startsWithNullText = $(".selector").igGridFiltering("option", "locale").startsWithNullText; //Set $(".selector").igGridFiltering("option", "locale", {startsWithNullText : "Starts With"});
-
thisMonthLabel
- Type:
- string
- Default:
- ""
'This Month' label that is used for the predefined filtering conditions in the filter dropdowns.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { thisMonthLabel : "This Month"} } ] }); //Get var thisMonthLabel = $(".selector").igGridFiltering("option", "locale").thisMonthLabel; //Set $(".selector").igGridFiltering("option", "locale", {thisMonthLabel : "This Month"});
-
thisYearLabel
- Type:
- string
- Default:
- ""
'This Year' label that is used for the predefined filtering conditions in the filter dropdowns.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { thisYearLabel : "This Year"} } ] }); //Get var thisYearLabel = $(".selector").igGridFiltering("option", "locale").thisYearLabel; //Set $(".selector").igGridFiltering("option", "locale", {thisYearLabel : "This Year"});
-
todayLabel
- Type:
- string
- Default:
- ""
'Today' label that is used for the predefined filtering conditions in the filter dropdowns.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { todayLabel : "Today"} } ] }); //Get var todayLabel = $(".selector").igGridFiltering("option", "locale").todayLabel; //Set $(".selector").igGridFiltering("option", "locale", {todayLabel : "Today"});
-
tooltipTemplate
- Type:
- string
- Default:
- ""
Custom tooltip template for the filter button, when a filter is applied.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { tooltipTemplate : "${condition} filter applied"} } ] }); //Get var tooltipTemplate = $(".selector").igGridFiltering("option", "locale").tooltipTemplate; //Set $(".selector").igGridFiltering("option", "locale", {tooltipTemplate : "${condition} filter applied"});
-
trueLabel
- Type:
- string
- Default:
- ""
'True' label that is used for the predefined filtering conditions in the filter dropdowns.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { trueLabel : "True"} } ] }); //Get var trueLabel = $(".selector").igGridFiltering("option", "locale").trueLabel; //Set $(".selector").igGridFiltering("option", "locale", {trueLabel : "True"});
-
yesterdayLabel
- Type:
- string
- Default:
- ""
'Yesterday' label that is used for the predefined filtering conditions in the filter dropdowns.
Code Sample
//Initialize $(".selector").igGrid({ features: [ { name : "Filtering", locale: { yesterdayLabel : "Yesterday"} } ] }); //Get var yesterdayLabel = $(".selector").igGridFiltering("option", "locale").yesterdayLabel; //Set $(".selector").igGridFiltering("option", "locale", {yesterdayLabel : "Yesterday"});
-
mode
- Type:
- enumeration
- Default:
- null
Default is 'simple' for non-virtualized grids, and 'advanced' when virtualization is enabled.
Members
- simple
- Type:string
- Renders just a filter row.
- advanced
- Type:string
- Allows to configure multiple filters from a dialog - Excel style.
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", mode : "advanced" } ] }); // Get var filterMode = $(".selector").igGridFiltering("option", "mode"); // Set $(".selector").igGridFiltering("option", "mode", "advanced");
-
nullTexts
Removed- Type:
- string
- Default:
- "{}"
This option has been removed as of 2017.2 Volume release.
List of configurable and localized null texts that will be used for the filter editors. Use option locale.Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", nullTexts: { contains: "Contains...", doesNotContain: "Does not contain...", doesNotEqual: "Does not equal...", empty: "Empty", endsWith: "Ends with..." //... } } ] }); // Get var filteringNullTexts = $(".selector").igGridFiltering("option", "nullTexts"); // Set $(".selector").igGridFiltering("option", "nullTexts", { contains: "Contains...", doesNotContain: "Does not contain...", doesNotEqual: "Does not equal...", empty: "Empty", endsWith: "Ends with..." //... });
-
after
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
-
contains
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
-
empty
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
-
equals
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
-
greaterThan
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
-
greaterThanOrEqualTo
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
-
lastYear
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
-
nextMonth
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
-
null
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
-
on
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
-
startsWith
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
-
thisMonth
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
-
persist
- Type:
- bool
- Default:
- true
Enables/disables filtering persistence between states.
Code Sample
// Initialize $(".selector").igGrid({ features : [ { name: "Filtering", persist: false } ] }); // Get var persist = $(".selector").igGridFiltering("option", "persist"); // Set $(".selector").igGridFiltering("option", "persist", false);
-
regional
Inherited- Type:
- enumeration
- Default:
- en-US
Set/Get the regional setting for the widget.
Code Sample
//Initialize $(".selector").igGridFiltering({ regional: "ja" }); // Get var regional = $(".selector").igGridFiltering("option", "regional"); // Set $(".selector").igGridFiltering("option", "regional", "ja");
-
renderFC
- Type:
- bool
- Default:
- true
Render in Feature Chooser.
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", renderFC: false } ] }); // Get var filteringRenderFC = $(".selector").igGridFiltering("option", "renderFC"); // Set $(".selector").igGridFiltering("option", "renderFC", false);
-
renderFilterButton
- Type:
- bool
- Default:
- true
Enable/disable filter button visibility. If false, no filter dropdown buttons will be rendered and a predefined list of filters will not be rendered for the columns.
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", renderFilterButton : false } ] }); // Get var showButton = $(".selector").igGridFiltering("option", "renderFilterButton"); // Set $(".selector").igGridFiltering("option", "renderFilterButton", false);
-
showEmptyConditions
- Type:
- bool
- Default:
- false
Enable/disable empty condition visibility in the filter. If true, shows empty and not empty filtering conditions in the dropdowns.
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", showEmptyConditions : true } ] }); // Get var showConditions = $(".selector").igGridFiltering("option", "showEmptyConditions"); // Set $(".selector").igGridFiltering("option", "showEmptyConditions", true);
-
showNullConditions
- Type:
- bool
- Default:
- false
Enable/disable visibility of null and not null filtering conditions in the dropdowns. If true, shows null and not null filtering conditions in the dropdowns.
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", showNullConditions : true } ] }); // Get var showConditions = $(".selector").igGridFiltering("option", "showNullConditions"); // Set $(".selector").igGridFiltering("option", "showNullConditions", true);
-
tooltipTemplate
Removed- Type:
- string
- Default:
- ""
This option has been removed as of 2017.2 Volume release.
Custom tooltip template for the filter button, when a filter is applied. Use option locale.tooltipTemplate.Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", tooltipTemplate: "${condition} filter applied" } ] }); // Get var template = $(".selector").igGridFiltering("option", "tooltipTemplate"); // Set $(".selector").igGridFiltering("option", "tooltipTemplate", "${condition} filter applied");
-
type
- Type:
- enumeration
- Default:
- null
Type of filtering. Delegates all filtering functionality to the $.ig.DataSource.
Members
- remote
- Type:string
- Filtering is performed by a remote end-point.
- local
- Type:string
- Filtering is performed locally by the $.ig.DataSource.
Code Sample
// Initialize $(".selector").igGrid({ features: [ { name : "Filtering", type : "local" } ] }); // Get var filterType = $(".selector").igGridFiltering("option", "type");
For more information on how to interact with the Ignite UI controls' events, refer to
Using Events in Ignite UI.
-
dataFiltered
- Cancellable:
- false
Event fired after the filtering has been executed and results are rendered.
-
evtType: Event
JQuery event object.
-
uiType: Object
-
ownerType: Object
Gets reference to GridFiltering.
-
owner.gridType: Object
Gets reference to the grid.
-
columnIndexType: Number
Gets the column index. Applicable only when filtering mode is "simple".
-
columnKeyType: String
Gets the column key. Applicable only when filtering mode is "simple".
-
expressionsType: Array
Gets the filtered expressions.
-
Code Sample
//Delegate $(document).delegate(".selector", "iggridfilteringdatafiltered", function (evt, ui) { //return column key ui.columnKey; //return column index ui.columnIndex; //return reference to igGridFiltering ui.owner; //return reference to igGrid ui.owner.grid; //return filtering expressions from the data source ui.expressions; }); //Initialize $(".selector").igGrid({ features: [ { name : "Filtering", dataFiltered: function (evt, ui) {...} } ] });
-
dataFiltering
- Cancellable:
- true
Event fired before a filtering operation is executed (remote request or local).
Return false in order to cancel filtering operation.-
evtType: Event
JQuery event object.
-
uiType: Object
-
ownerType: Object
Gets reference to GridFiltering.
-
owner.gridType: Object
Gets reference to the grid.
-
columnIndexType: Number
Gets the column index. Applicable only when filtering mode is "simple".
-
columnKeyType: String
Gets the column key. Applicable only when filtering mode is "simple".
-
newExpressionsType: Array
Gets the filtering expressions. Filtering expressions could be changed in this event handler and after that data binding is applied. In this way the user could control filtering more easily before applying data-binding.
-
Code Sample
//Delegate $(document).delegate(".selector", "iggridfilteringdatafiltering", function (evt, ui) { //return column key ui.columnKey; //return column index ui.columnIndex; //return reference to igGridFiltering ui.owner; //return reference to igGrid ui.owner.grid; //return the new filtering expressions that are going to be applied to the data source ui.newExpressions; }); //Initialize $(".selector").igGrid({ features: [ { name : "Filtering", dataFiltering: function (evt, ui) {...} } ] });
-
dropDownClosed
- Cancellable:
- false
Event fired after a filter column dropdown is completely closed.
Code Sample
//Delegate $(document).delegate(".selector", "iggridfilteringdropdownclosed", function (evt, ui) { //return reference to igGridFiltering ui.owner; //return reference to igGrid ui.owner.grid; //return dropdown html element in the DOM ui.dropDown; }); //Initialize $(".selector").igGrid({ features: [ { name : "Filtering", dropDownClosed: function (evt, ui) {...} } ] });
-
dropDownClosing
- Cancellable:
- true
Event fired before the filter dropdown starts closing.
Return false in order to cancel dropdown closing.Code Sample
//Delegate $(document).delegate(".selector", "iggridfilteringdropdownclosing", function (evt, ui) { //return reference to igGridFiltering ui.owner; //return reference to igGrid ui.owner.grid; //return dropdown html element in the DOM ui.dropDown; }); //Initialize $(".selector").igGrid({ features: [ { name : "Filtering", dropDownClosing: function (evt, ui) {...} } ] });
-
dropDownOpened
- Cancellable:
- false
Event fired after the filter dropdown is opened for a specific column.
Code Sample
//Delegate $(document).delegate(".selector", "iggridfilteringdropdownopened", function (evt, ui) { //return reference to igGridFiltering ui.owner; //return reference to igGrid ui.owner.grid; //return dropdown html element in the DOM ui.dropDown; }); //Initialize $(".selector").igGrid({ features: [ { name : "Filtering", dropDownOpened: function (evt, ui) {...} } ] });
-
dropDownOpening
- Cancellable:
- true
Event fired before the filter dropdown is opened for a specific column.
Return false in order to cancel dropdown opening.Code Sample
//Delegate $(document).delegate(".selector", "iggridfilteringdropdownopening", function (evt, ui) { //return reference to igGridFiltering ui.owner; //return reference to igGrid ui.owner.grid; //return dropdown html element in the DOM ui.dropDown; }); //Initialize $(".selector").igGrid({ features: [ { name : "Filtering", dropDownOpening: function (evt, ui) {...} } ] });
-
filterDialogClosed
- Cancellable:
- false
Event fired after the advanced filter dialog has been closed.
Code Sample
//Delegate $(document).delegate(".selector", "iggridfilteringfilterdialogclosed", function (evt, ui) { //return reference to igGridFiltering ui.owner; //return reference to igGrid ui.owner.grid; }); //Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDialogClosed: function (evt, ui) {...} } ] });
-
filterDialogClosing
- Cancellable:
- true
Event fired before the advanced filter dialog is closed.
Return false in order to cancel filtering dialog closing.Code Sample
//Delegate $(document).delegate(".selector", "iggridfilteringfilterdialogclosing", function (evt, ui) { //return reference to igGridFiltering ui.owner; //return reference to igGrid ui.owner.grid; }); //Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDialogClosing: function (evt, ui) {...} } ] });
-
filterDialogContentsRendered
- Cancellable:
- false
Event fired after the contents of the advanced filter dialog are rendered.
Code Sample
//Delegate $(document).delegate(".selector", "iggridfilteringfilterdialogcontentsrendered", function (evt, ui) { //return reference to igGridFiltering ui.owner; //return reference to igGrid ui.owner.grid; //return dialog html element in the DOM ui.dialogElement; }); //Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDialogContentsRendered: function (evt, ui) {...} } ] });
-
filterDialogContentsRendering
- Cancellable:
- true
Event fired before the contents of the advanced filter dialog are rendered.
Return false in order to cancel filtering dialog rendering.Code Sample
//Delegate $(document).delegate(".selector", "iggridfilteringfilterdialogcontentsrendering", function (evt, ui) { //return reference to igGridFiltering ui.owner; //return reference to igGrid ui.owner.grid; //return dialog html element in the DOM ui.dialogElement; }); //Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDialogContentsRendering: function (evt, ui) {...} } ] });
-
filterDialogFilterAdded
- Cancellable:
- false
Event fired after a filter row is added to the advanced filter dialog.
Code Sample
//Delegate $(document).delegate(".selector", "iggridfilteringfilterdialogfilteradded", function (evt, ui) { //return reference to igGridFiltering ui.owner; //return reference to igGrid ui.owner.grid; //return filters table row element in the DOM ui.filter; }); //Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDialogFilterAdded: function (evt, ui) {...} } ] });
-
filterDialogFilterAdding
- Cancellable:
- true
Event fired before a filter row is added to the advanced filter dialog.
Return false in order to cancel filter adding to the advanced filtering dialog.Code Sample
//Delegate $(document).delegate(".selector", "iggridfilteringfilterdialogfilteradding", function (evt, ui) { //return reference to igGridFiltering ui.owner; //return reference to igGrid ui.owner.grid; //return filters table body element in the DOM ui.filtersTableBody; }); //Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDialogFilterAdding: function (evt, ui) {...} } ] });
-
filterDialogFiltering
- Cancellable:
- true
Event fired when the OK button in the advanced filter dialog is pressed.
Code Sample
//Delegate $(document).delegate(".selector", "iggridfilteringfilterdialogfiltering", function (evt, ui) { //return reference to igGridFiltering ui.owner; //return reference to igGrid ui.owner.grid; //return dialog html element in the DOM ui.dialog; }); //Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDialogFiltering: function (evt, ui) {...} } ] });
-
filterDialogMoving
- Cancellable:
- true
Event fired every time the advanced filter dialog changes its position.
-
evtType: Event
JQuery event object.
-
uiType: Object
-
ownerType: Object
Gets reference to GridFiltering.
-
owner.gridType: Object
Gets reference to the grid.
-
dialogType: jQuery
Gets reference to filtering dialog DOM element.
-
originalPositionType: Object
Gets the original position of the groupby dialog div as { top, left } object, relative to the page.
-
positionType: Object
Gets the current position of the groupby dialog div as { top, left } object, relative to the page.
-
Code Sample
//Delegate $(document).delegate(".selector", "iggridfilteringfilterdialogmoving", function (evt, ui) { //return reference to igGridFiltering ui.owner; //return reference to igGrid ui.owner.grid; //return dialog html element in the DOM ui.dialog; }); //Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDialogMoving: function (evt, ui) {...} } ] });
-
filterDialogOpened
- Cancellable:
- false
Event fired after the advanced filter dialog is already opened.
Code Sample
//Delegate $(document).delegate(".selector", "iggridfilteringfilterdialogopened", function (evt, ui) { //return reference to igGridFiltering ui.owner; //return reference to igGrid ui.owner.grid; //return dialog html element in the DOM ui.dialog; }); //Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDialogOpened: function (evt, ui) {...} } ] });
-
filterDialogOpening
- Cancellable:
- true
Event fired before the advanced filtering dialog is opened.
Return false in order to cancel filter dialog opening.Code Sample
//Delegate $(document).delegate(".selector", "iggridfilteringfilterdialogopening", function (evt, ui) { //return reference to igGridFiltering ui.owner; //return reference to igGrid ui.owner.grid; //return dialog html element in the DOM ui.dialog; }); //Initialize $(".selector").igGrid({ features: [ { name : "Filtering", filterDialogOpening: function (evt, ui) {...} } ] });
-
changeLocale
- .igGridFiltering( "changeLocale" );
Changes the all locales into the widget element to the language specified in options.language
Note that this method is for rare scenarios, see language or locale option setter.Code Sample
$(".selector").igGridFiltering("changeLocale");
-
changeRegional
- .igGridFiltering( "changeRegional" );
Changes the the regional settings of widget element to the language specified in options.regional
Note that this method is for rare scenarios, use regional option setter.Code Sample
$(".selector").igGridFiltering("changeRegional");
-
destroy
- .igGridFiltering( "destroy" );
Destroys the filtering widget - remove fitler row, unbinds events, returns the grid to its previous state.
Code Sample
$(".selector").igGridFiltering("destroy");
-
filter
- .igGridFiltering( "filter", expressions:array, [updateUI:bool] );
Applies filtering programmatically and updates the UI by default.
- expressions
- Type:array
- An array of filtering expressions, each one having the format {fieldName: , expr: , cond: , logic: } where fieldName is the key of the column, expr is the actual expression string with which we would like to filter, logic is 'AND' or 'OR', and cond is one of the following strings: "equals", "doesNotEqual", "contains", "doesNotContain", "greaterThan", "lessThan", "greaterThanOrEqualTo", "lessThanOrEqualTo", "true", "false", "null", "notNull", "empty", "notEmpty", "startsWith", "endsWith", "today", "yesterday", "on", "notOn", "thisMonth", "lastMonth", "nextMonth", "before", "after", "thisYear", "lastYear", "nextYear". The difference between the empty and null filtering conditions is that empty includes null, NaN, and undefined, as well as the empty string.
- updateUI
- Type:bool
- Optional
- specifies whether the filter row should be also updated once the grid is filtered.
Code Sample
/* Expressions is an array of individual filtering expressions that the grid uses when filtering. If you only want to execute one filter criteria, then you add a single filtering expression to the array, otherwise you can add additional constraints to the filter by adding more expressions into the array. For example: [{ fieldname: "title", expr: "introduction", cond: "contains", logic: "OR" }, { fieldname: "description", expr: "introduction", cond: "contains", logic: "OR" }] Using the expressions above the applied filter returns records in the grid where either the "title" or "description" fields have values that contain the string "introduction". Note: The following conditions (grouped by data type) are available to perform filtering on the grid: - String - startsWith - endsWith - contains - doesNotContain - equals - doesNotEqual - null - notNull - empty - notEmpty - Number - equals - doesNotEqual - greaterThan - lessThan - greaterThanOrEqualTo - lessThanOrEqualTo - null - notNull - empty - notEmpty - Boolean - true - false - null - notNull - empty - notEmpty - Date - on - notOn - after - before - today - yesterday - thisMonth - lastMonth - nextMonth - thisYear - lastYear - nextYear - null - notNull - empty - notEmpty - Object - null - notNull - empty - notEmpty The difference between the "empty" and "null" filtering conditions is that "empty" includes "null", "NaN", "undefined" and empty strings. Note: Available values for "logic" are "OR" and "AND". The default logic is "AND". */ $(".selector").igGridFiltering("filter", ([{fieldName: "Name", expr: "Adjustable Race", cond: "equals", logic: "OR"}]));
-
getFilteringMatchesCount
- .igGridFiltering( "getFilteringMatchesCount" );
- Return Type:
- number
- Return Type Description:
- Count of filtered records.
Returns the count of data records that match filtering conditions.
Code Sample
var matchesCount = $(".selector").igGridFiltering("getFilteringMatchesCount");
-
requiresFilteringExpression
- .igGridFiltering( "requiresFilteringExpression", filterCondition:string );
- Return Type:
- bool
- Return Type Description:
- if false then filterCondition does not require filtering expression.
Check whether filterCondition requires or not filtering expression - e.g. if filterCondition is "lastMonth", "thisMonth", "null", "notNull", "true", "false", etc. then filtering expression is NOT required.
- filterCondition
- Type:string
- filtering condition - e.g. "true", "false", "yesterday", "empty", "null", etc.
Code Sample
$(".selector").igGridFiltering("requiresFilteringExpression", "yesterday");
-
toggleFilterRowByFeatureChooser
- .igGridFiltering( "toggleFilterRowByFeatureChooser", event:string );
Toggle filter row when mode is simple or advancedModeEditorsVisible is true. Otherwise show/hide advanced dialog.
- event
- Type:string
- Column key.
Code Sample
$(".buttonSelector").igButton({ labelText: $(".buttonSelector").val(), click: function (event) { $(".gridSelector").igGridFiltering("toggleFilterRowByFeatureChooser", event); } });
-
ui-widget-overlay ui-iggrid-blockarea
- Classes applied to the filtering block area, when the advanced filter dialog is opened and the area behind it is grayed out (that's the block area).
-
ui-icon ui-iggrid-icon-advanced-filter
- Classes applied to the feature chooser icon when filter shows advanced dialog.
-
ui-iggrid-filterbutton ui-corner-all ui-icon ui-icon-triangle-1-s
- Classes applied to every filtering dropdown button.
-
ui-iggrid-filterbuttonactive ui-state-active
- Classes applied to the filter button when it is selected.
-
ui-iggrid-filterbutton ui-iggrid-filterbuttonadvanced ui-icon ui-icon-search
- Classes applied to the button when mode = advanced. This also applies to the button when it's rendered in the header (which is the default behavior).
-
ui-iggrid-filterbuttonadvancedactive ui-state-active
- Classes applied on the advanced button when it is selected.
-
ui-iggrid-filterbuttonadvanceddisabled ui-state-disabled
- Classes applied on the advanced button when it is disabled.
-
ui-iggrid-filterbuttonadvancedfocus ui-state-focus
- Classes applied on the advanced button when it has focus.
-
ui-iggrid-filterbuttonadvancedhover ui-state-hover
- Classes applied on the advanced button when it is hovered.
-
ui-iggrid-filterbuttonright ui-iggrid-filterbuttonadvanced ui-icon ui-icon-search
- Classes applied to the advanced filtering button when it is rendered on the right.
-
ui-iggrid-filterbuttonbool
- Classes applied to the filter button when a boolean filter is applied for the column (default).
-
ui-iggrid-filterbuttondate
- Classes applied to the filter button when a date filter is defined for the column.
-
ui-iggrid-filterbuttondisabled ui-state-disabled
- Classes applied to the filtering button when it is disabled.
-
ui-iggrid-filterbuttonfocus ui-state-focus
- Classes applied to the filter button when it has focus but is not selected.
-
ui-iggrid-filterbuttonhover ui-state-hover
- Classes applied to the filter button when it is hovered.
-
ui-iggrid-filterbuttonnumber
- Classes applied to the filter button when a number filter is applied for the column (default).
-
ui-iggrid-filterbuttonstring
- Classes applied to the filter button when a string filter is applied for the column (default).
-
ui-iggrid-filtercell
- Classes applied to every filter cell TH.
-
ui-iggrid-filtereditor
- Classes applied to every filter editor element (igEditor).
-
ui-dialog ui-draggable ui-resizable ui-iggrid-dialog ui-widget ui-widget-content ui-corner-all
- Classes applied to the filter dialog element.
-
ui-iggrid-filterdialogaddbuttoncontainer ui-helper-reset
- Classes applied to the filter dialog add button.
-
ui-iggrid-filterdialogaddcondition
- Classes applied to the filter dialog add condition area.
-
ui-iggrid-filterdialogaddconditionlist
- Classes applied to the filter dialog add condition SELECT dropdown.
-
ui-iggrid-filterdialogclearall
- Classes applied to the filter dialog "Clear All" button.
-
ui-icon ui-icon-closethick
- Classes applied to the "X" button used to remove filters from the filters table.
-
ui-iggrid-filtertable ui-helper-reset
- Classes applied to the filter dialog filters table.
-
ui-dialog-titlebar ui-iggrid-filterdialogcaption ui-widget-header ui-corner-all ui-helper-reset ui-helper-clearfix
- Classes applied to the filter dialog header caption area.
-
ui-dialog-title
- Class applied to the filter dialog header caption title.
-
ui-dialog-buttonpane ui-widget-content ui-helper-clearfix ui-iggrid-filterdialogokcancelbuttoncontainer
- Classes applied to the filter dialog OK and Cancel buttons.
-
ui-iggrid-filterdd
- Classes applied to the DIV which wraps the dropdown UL.
-
ui-menu ui-widget ui-widget-content ui-iggrid-filterddlist ui-corner-all
- Classes applied to the UL filter dropdown list.
-
ui-iggrid-filterddlistitem
- Classes applied to each filter dropdown list item (LI).
-
ui-iggrid-filterddlistitemactive ui-state-active
- Classes applied to the list item when it is selected.
-
ui-iggrid-filterddlistitemadvanced
- Class applied to the list item that holds the Advanced button, if options are configured such that editors are shown when mode = "advanced".
-
ui-iggrid-filterddlistitemclear
- Classes applied to the "clear" filter list item.
-
ui-iggrid-filterddlistitemhover ui-state-hover
- Classes applied to the list item when it is hovered.
-
ui-iggrid-filterddlistitemcontainer
- Classes applied to the element that holds the text in every filter list item (LI).
-
ui-iggrid-filterddlistitemicons ui-state-default
- Classes applied to the list item when filtering icons are visible for it.
-
ui-iggrid-filtericon
- Classes applied to every filter dropdown list item's image icon area.
-
ui-iggrid-filtericonafter
- Classes applied to the item icon's span when the item holds an after condition.
-
ui-iggrid-filtericonbefore
- Classes applied to the item icon's span when the item holds a before condition.
-
ui-iggrid-filtericonclear
- Classes applied to the item icon's span when the item holds a clear condition.
-
ui-iggrid-filtericoncontainer
- Classes applied to the item icon's container element.
-
ui-iggrid-filtericoncontains
- Classes applied to the item icon's span when the item holds a contains condition.
-
ui-iggrid-filtericondoesnotcontain
- Classes applied to the item icon's span when the item holds a doesNotContain condition.
-
ui-iggrid-filtericondoesnotequal
- Classes applied to the item icon's span when the item holds a doesNotEqual condition.
-
ui-iggrid-filtericonendswith
- Classes applied to the item icon's span when the item holds an endsWith condition.
-
ui-iggrid-filtericonequals
- Classes applied to the item icon's span when the item holds a contains condition.
-
ui-iggrid-filtericonfalse
- Classes applied to the item icon's span when the item holds a false condition.
-
ui-iggrid-filtericongreaterthan
- Classes applied to the item icon's span when the item holds a greaterThan condition.
-
ui-iggrid-filtericongreaterthanorequalto
- Classes applied to the item icon's span when the item holds a greaterThanOrEqualTo condition.
-
ui-iggrid-filtericonlastmonth
- Classes applied to the item icon's span when the item holds a lastMonth condition.
-
ui-iggrid-filtericonlastyear
- Classes applied to the item icon's span when the item holds a lastYear condition.
-
ui-iggrid-filtericonlessthan
- Classes applied to the item icon's span when the item holds a lessThan condition.
-
ui-iggrid-filtericonlessthanorequalto
- Classes applied to the item icon's span when the item holds a lessThanOrEqualTo condition.
-
ui-iggrid-filtericonnextmonth
- Classes applied to the item icon's span when the item holds a nextMonth condition.
-
ui-iggrid-filtericonnextyear
- Classes applied to the item icon's span when the item holds a nextYear condition.
-
ui-iggrid-filtericonnoton
- Classes applied to the item icon's span when the item holds a notOn condition.
-
ui-iggrid-filtericonon
- Classes applied to the item icon's span when the item holds an on condition.
-
ui-iggrid-filtericonstartswith
- Classes applied to the item icon's span when the item holds a startsWith condition.
-
ui-iggrid-filtericonthismonth
- Classes applied to the item icon's span when the item holds a thisMonth condition.
-
ui-iggrid-filtericonthisyear
- Classes applied to the item icon's span when the item holds a thisYear condition.
-
ui-iggrid-filtericontoday
- Classes applied to the item icon's span when the item holds a today condition.
-
ui-iggrid-filtericontrue
- Classes applied to the item icon's span when the item holds a true condition.
-
ui-iggrid-filtericonyesterday
- Classes applied to the item icon's span when the item holds a yesterday condition.
-
ui-iggrid-filterrow ui-widget
- Classes applied to the filter row TR in the headers table.